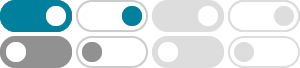
Array of Dates in Java - Stack Overflow
Nov 26, 2009 · you can use an array of java.util.Date (API docs are here) Date[] dates = new Date[] { new Date(), new Date(), }; You can create an array of any object type in java - all reference and primitive types
Java Date and Time - W3Schools
Java does not have a built-in Date class, but we can import the java.time package to work with the date and time API. The package includes many date and time classes. For example: If you don't know what a package is, read our Java Packages Tutorial. To display the current date, import the java.time.LocalDate class, and use its now() method:
How to sort dates in a string array in Java? - Stack Overflow
The better way would be to convert the String array to an array of Date. Date[] arrayOfDates = new Date[dates.length]; for (int index = 0; index < dates.length; index++) { arrayOfDates[index] = sdf.parse(dates[index]); } Then sort the arrayOfDates and if you need to, use the SimpleDateFormat to format the results back to the dates array...
java - Sort objects in ArrayList by date? - Stack Overflow
public class A { private Date dateTime; public Date getDateTime() { return dateTime; } .... other variables } And let's say you have a list of A objects as List<A> aList, you can easily sort it with Java 8's stream API (snippet below): import java.util.Comparator; import java.util.stream.Collectors; ...
Sorting Objects in a List by Date - Baeldung
Apr 3, 2025 · In this article, we explored how to sort a Java Collection by Date object in both ascending and descending modes. We also briefly saw the Java 8 lambda features that are useful in sorting and help in making the code concise.
Date class in Java (With Examples) - GeeksforGeeks
Jan 2, 2019 · The class Date represents a specific instant in time, with millisecond precision. The Date class of java.util package implements Serializable, Cloneable and Comparable interface. It provides constructors and methods to deal with date and time with java. Constructors. Date(): Creates date object representing current date and time.
Sorting the date in ascending order in java - Wisdom Overflow
Nov 1, 2018 · Sorting the date given by the user in a day, month, year order. Sort them in ascending order and display them. First get the list of dates from the user as a string. Create an array of objects for Date class and dynamically allocate memory. Create an object for SimpleDateFormat class with the format as “dd-MM-yyyy”.
Finding Max and Min Date in List Using Streams - Baeldung
Apr 3, 2025 · In this article, we’ll explore how to find the maximal and minimal date in a list of those objects using Streams. 2. Example Setup. Java’s original Date API is still widely used, so we’ll showcase an example using it. However, since Java 8, LocalDate was introduced, and most Date methods were deprecated.
How to Sort List by Date in Java 8 - JavaTechOnline
Mar 31, 2025 · Let’s examine various possible approaches of Java 8 to sort a list by date. These are: 1) Using Lambda Expression. 2) Using Method References. 3) Using Stream API Methods. In order to implement sorting a List by Date, let’s first create a POJO which will have a …
Parsing Date Strings with Varying Formats - Baeldung
Jan 8, 2024 · In this tutorial, we’ll demonstrate some options that we have for parsing different patterns of dates. First, we’ll try to solve parsing problems using standard Java libraries: SimpleDateFormat and DateTimeFormatterBuilder. Then, we’ll examine third-party libraries Apache Commons DateUtils and Joda Time. 2. Using SimpleDateFormat.