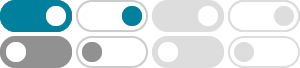
How to find cube root using Python? - Stack Overflow
Jan 18, 2015 · Since Python 3.11, which will be released in a couple months, you can just use math.cbrt(x), obviously having imported math first with import math. It will also cover the negative case, and will be more optimized than x ** (1 / 3). You could use x ** (1. / 3) to compute the (floating-point) cube root of x.
Python Program for Find cubic root of a number - GeeksforGeeks
Jul 27, 2023 · This method uses the iterative method of computing the cubic root of a number. The idea is to start with an initial guess and improve the guess iteratively until the difference between the guess and the actual cubic root is small enough. This method is known as the Babylonian method or Heron’s method.
5 Best Ways to Calculate the Cube Root of a Given Number in Python
Feb 27, 2024 · For fans of Python’s concise syntax, a lambda function can be used to create an anonymous function to compute the cube root. It’s ideal for one-off calculations without the need for defining a full function. Here’s an example: cube_root = lambda x: x ** (1/3) print('The cube root of 125 is', cube_root(125)) Output: The cube root of 125 is 5.0
How to Calculate Cube Root in Python - Delft Stack
Feb 2, 2024 · One straightforward approach to calculate the cube root of an integer or a float variable in Python is by using the exponent symbol **. The exponent symbol ** is an operator that raises a number to a specified power. To compute the cube root, we can leverage this symbol by setting the power to 1/3. The syntax is as follows:
How to get a cube root in Python - Stack Overflow
In Python, you may find floating cube root by: >>> def get_cube_root(num): ... return num ** (1. / 3) ... >>> get_cube_root(27) 3.0 In case you want more generic approach to find nth root, you may use below code which is based on Newton's method:
Calculating Cube Roots in Python: A Comprehensive Guide
Jan 23, 2025 · This blog post will delve into the various methods of taking the cube root in Python, covering fundamental concepts, usage, common practices, and best practices. Table of Contents. Fundamental Concepts of Cube Roots; Using the math Module to Calculate Cube Roots. Basic Usage; Handling Negative Numbers; Using Exponentiation to Calculate Cube Roots
Mastering Cube Root Calculation in Python – Step-by-Step Guide
When working with mathematical calculations in Python, understanding how to compute the cube root of a number is essential. The cube root refers to finding the value that, when raised to the power of three, yields the original number.
Find cube root of a number in Python - CodeSpeedy
Feb 11, 2024 · We can define a function for cube root. When a user inputs a number for cube root, it will automatically return the cube root of the number. def cube_root(x): return x**(1/3) print(cube_root(27))
Mastering Square Root and Cube Root in Python - CodeRivers
Apr 7, 2025 · This blog post will explore the concepts, usage methods, common practices, and best practices related to square root and cube root operations in Python. Table of Contents. Fundamental Concepts of Square Root and Cube Root; Calculating Square Root in Python. Using the math Module; Using the numpy Library; Calculating Cube Root in Python. Manual ...
Python cbrt() Method - Online Tutorials Library
The Python math.cbrt() method is used to calculate the cube root of a given number. Mathematically, the cube root method, denoted as ∛x, is a mathematical operation that finds a number which, when multiplied by itself twice, gives the original number x.
- Some results have been removed