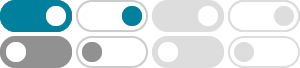
python - How do you round UP a number? - Stack Overflow
May 5, 2017 · Use math.ceil to round up: >>> import math >>> math.ceil(5.4) 6.0 NOTE: The input should be float. If you need an integer, call int to convert it: >>> int(math.ceil(5.4)) 6 BTW, use math.floor to round down and round to round to nearest integer.
Python Round to Int – How to Round Up or Round Down to the …
May 24, 2022 · When working with float values (numbers with decimal values) in our Python program, we might want to round them up or down, or to the nearest whole number. In this article, we'll see some built-in functionalities that let us round numbers in Python. And we'll see how to use them with some examples. We'll start with the round() function. By ...
Round to 5 (or other number) in Python - Stack Overflow
Jul 7, 2021 · It's an adaptation of the simplest solution for rounding down to the next lower multiple of a number: def round_to_nearest(num, base=5): num += base // 2 return num - (num % base) The round down recipe it's based on is just: def round_down(num, base=5): return num - …
How to Round Numbers in Python? - GeeksforGeeks
Dec 8, 2023 · In Python, there is a built-in round () function that rounds off a number to the given number of digits. The function round () accepts two numeric arguments, n, and n digits, and then returns the number n after rounding it to n digits.
Python 2.7: rounding down instead of rounding up
Jun 28, 2015 · So your function is currently not rounding up but rounding to the nearest one. What you can do to always round down at two decimals is . int(100*x)/100.0 which always rounds to zero, or. math.floor(100*x)/100.0 to always round down. So you can have: Normal: def __str__(self): return'x = %.2f,y = %.2f'%(self.x,self.y) Always round to zero:
How to Round Up a Number in Python - DataCamp
Jul 22, 2024 · If you want to round down instead of rounding up in Python, you can use the math.floor() function. While math.ceil() rounds a number up to the nearest integer, math.floor() rounds a number down to the nearest integer.
How to Round Numbers in Python? - Python Guides
Jan 15, 2025 · Python provides built-in functions and modules that make rounding numbers easy. Let us see some important methods to achieve this task. 1. Use the Built-in round ()Function. Python has a built-in round() function that takes two arguments: the number to be rounded and an optional number of decimal places. The basic syntax is as follows:
Rounding Up in Python: A Comprehensive Guide - CodeRivers
2 days ago · In Python, rounding numbers is a common operation in various mathematical and data processing tasks. Rounding up specifically refers to the process of increasing a number to the next whole number or a specified decimal place. This blog post will explore different ways to round up numbers in Python, covering the fundamental concepts, usage methods, common practices, and best practices. Whether ...
Python round up practical examples [5 Methods] - GoLinuxCloud
Nov 16, 2021 · Python round up methods, math.ceil() method, floor division method, simple arithmetic method, and nampy.ceil() method to round up a number in Python.
Top 10 Effective Methods to Round UP a Number in Python
Dec 5, 2024 · Below, we explore multiple methods to round up numbers in Python, along with practical examples. Method 1: Using the math.ceil() Function. The math module provides a straightforward function, math.ceil(), that rounds a floating-point number UP to the nearest whole number. Here’s how it works: number = 5.4 rounded_value = math.ceil(number)