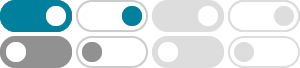
Binary Tree in Python - GeeksforGeeks
Feb 27, 2025 · A Binary search tree is a binary tree where the values of the left sub-tree are less than the root node and the values of the right sub-tree are greater than the value of the root node. In this article, we will discuss the binary search tree in Python.
python - How to implement a binary tree? - Stack Overflow
Apr 8, 2010 · A Tree is an even more general case of a Binary Tree where each node can have an arbitrary number of children. Typically, each node has a 'children' element which is of type list/array. Now, to answer the OP's question, I am including …
Tree Traversal Techniques in Python - GeeksforGeeks
Jan 22, 2024 · Tree sort is a sorting algorithm that builds a Binary Search Tree (BST) from the elements of the array to be sorted and then performs an in-order traversal of the BST to get the elements in sorted order. In this article, we will learn about the basics of Tree Sort along with its implementation in Py
Binary Trees in Python: Implementation and Examples
Jun 19, 2024 · The implementation section provided a step-by-step guide to creating a binary tree in Python. We covered the insertion process, different traversal methods (inorder, preorder, postorder, and BFS), and advanced operations such as searching and deleting nodes.
Python Binary Tree - Online Tutorials Library
Learn about Python binary trees, their properties, types, and implementation details. Explore how to create and manipulate binary tree structures in Python.
Binarytree Module in Python - GeeksforGeeks
Jan 10, 2023 · The binary search tree is a special type of tree data structure whose inorder gives a sorted list of nodes or vertices. In Python, we can directly create a BST object using binarytree module. bst() generates a random binary search tree and return its root node.
Binary Tree Traversal Algorithms in Python – Learn Programming
Mar 3, 2025 · The objective of this tutorial is to help you implement the three basic binary tree traversal algorithms (In-order, Pre-order, Post-order) using Python. By the end, you will understand how to traverse a binary tree and how each algorithm works.
Binary Tree Implementation in Python: A Comprehensive Guide
Apr 8, 2025 · In this blog, we have explored the fundamental concepts of binary tree implementation in Python. We have seen how to define nodes, create and manipulate trees, perform common operations like insertion, search, and …
Binary Trees in Python: A Comprehensive Guide - CodeRivers
Mar 17, 2025 · In Python, working with binary trees can be both efficient and elegant. This blog will explore the basic concepts of binary trees, how to implement them in Python, common operations, and best practices. A binary tree is a tree - like data structure where each node has at most two children.
Exploring Binary Trees in Python: Concepts, Usage, and Best …
Feb 10, 2025 · In Python, implementing and working with binary trees can be both straightforward and efficient. This blog post will delve into the fundamental concepts of binary trees in Python, explore their usage methods, discuss common practices, and provide best practices to help you master this essential data structure.