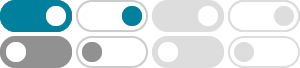
Binary Search (Recursive and Iterative) – Python - GeeksforGeeks
Feb 21, 2025 · Binary Search Algorithm is a searching algorithm used in a sorted array by repeatedly dividing the search interval in half. The idea of binary search is to use the information that the array is sorted and reduce the time complexity to O (log N). Below is the step-by-step algorithm for Binary Search:
Binary search in a Python list - Stack Overflow
Jul 13, 2016 · I am trying to perform a binary search on a list in python. List is created using command line arguments. User inputs the number he wants to look for in the array and he is returned the index of the
Binary Search (With Code) - Programiz
Binary Search is a searching algorithm for finding an element's position in a sorted array. In this tutorial, you will understand the working of binary search with working code in C, C++, Java, and Python.
Python Program For Binary Search (With Code) - Python Mania
To implement the binary search algorithm in Python, we need a sorted collection of elements and a target value to search for. Let’s start by writing a Python function for binary search.
Binary Search on ArrayList | Practice | GeeksforGeeks
Given a sorted integer arrayList and an integer element k. The task is to check if the element is present in the arrayList or not and return the index. Examples : Input: list[] = [1, 2, 3, 4, 6], k = 6 Output: 4 Explanation: Since, 6 is present
Binary Search in Python
Binary search is a searching algorithm that follows the divide and conquer approach to search for an element in a sorted array. In this blog, we will explore how to create a binary search in Python.
Python Program to Implement a Binary Search Algorithm
Jan 23, 2025 · Learn how to implement the binary search algorithm in Python with step-by-step examples. Understand binary search time complexity, recursive and iterative methods, and real-world use cases to boost your coding skills.
Binary Search in Python (Recursive and Iterative)
Today we are going to learn about the Binary Search Algorithm, it’s working, and will create a Project for binary search algorithm using Python and its modules.
Binary Search in Python: Concepts, Usage, and Best Practices
Jan 29, 2025 · Binary search is a powerful and efficient algorithm used for finding a target value within a sorted list. In Python, understanding and implementing binary search can significantly improve the performance of your code when dealing with large datasets.
How to Implement Binary Search in List in Python (2 Examples)
The aim of the binary search algorithm is to determine whether the target value exists in the list and, if it does, to find its index. The function we will implement needs two input parameters: a sorted list and a target value.