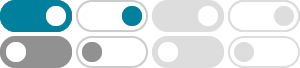
How do I assign a dictionary value to a variable in Python?
Nov 17, 2012 · The only way I could think to do it would be to assign a dictionary value to a variable, then assign the new value to the dictionary. Something like this: my_dictionary { 'foo' = 10, 'bar' = 20, } variable = my_dictionary['foo'] new_variable += variable my_dictionary['foo'] = …
python - How can I assign a value of a existing key in a dictionary ...
Nov 9, 2016 · dict={} for item in list: if item not in dict.keys(): dict[item] = None dict[item]=value If item is not present in dictionary's keys, just create one with None as starting value. Then, assign value to key (now You're sure it is there).
Add a key value pair to Dictionary in Python - GeeksforGeeks
Jan 23, 2025 · The task of adding a key-value pair to a dictionary in Python involves inserting new pairs or updating existing ones. This operation allows us to expand the dictionary by adding new entries or modify the value of an existing key.
How to Add Values to Dictionary in Python - GeeksforGeeks
Jan 23, 2025 · The task of adding values to a dictionary in Python involves inserting new key-value pairs or modifying existing ones. A dictionary stores data in key-value pairs, where each key must be unique. Adding values allows us to expand or update the dictionary’s contents, enabling dynamic manipulation of data.
python - How can I add new keys to a dictionary? - Stack Overflow
Jun 21, 2009 · You create a new key/value pair on a dictionary by assigning a value to that key d = {'key': 'value'} print(d) # {'key': 'value'} d['mynewkey'] = 'mynewvalue' print(d) # {'key': 'value', 'mynewkey': 'mynewvalue'}
6 Clever Ways to Assign Dictionary Values to Variables in Python
In this article, we will explore different ways of assigning values to variables from a dictionary in Python. 1) Assigning values to variables using bracket notation. One way to assign a value to a variable in Python is to use bracket notation to access the value of a key in a dictionary.
Python Dictionary: Guide To Work With Dictionaries In Python
Update Values in a Python Dictionary; Change a Key in a Dictionary in Python; Remove Multiple Keys from a Dictionary in Python; Create a Dictionary in Python Using a For Loop; Sum All Values in a Python Dictionary; Slice a Dictionary in Python; Save a Python Dictionary as a JSON File;
How to Assign Dictionary Keys and Values to Variables in Python
This guide explains various ways to assign dictionary keys and values to individual variables in Python. We'll cover direct access, using locals().update(), SimpleNamespace, and the less-recommended exec() method, discussing the use cases and trade-offs of each.
Python: Using variables as dictionary keys (basic and advanced …
Feb 12, 2024 · Starting with the basics, using variables as dictionary keys is straightforward. It involves defining a variable and then using it to assign a value within a dictionary. Output: This approach makes the code more readable and maintainable, as …
Assign a dictionary Key or Value to variable in Python
Apr 9, 2024 · Use bracket notation to assign a dictionary value to a variable, e.g. first = my_dict['first_name']. The left-hand side of the assignment is the variable's name and the right-hand side is the value. The code for this article is available on GitHub.
- Some results have been removed