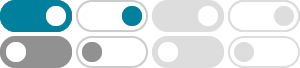
Creating class and objects - Programming - Arduino Forum
May 30, 2022 · A class (classname) contains functions and variables. An object is created from classname. Functions of the classname are applied on objects and then the functions are termed as methods. Example: (blinking of L (built-in LED of UNO) using class based codes
class - Using classes with the Arduino - Stack Overflow
Aug 2, 2011 · There is an excellent tutorial on how to create a library for the Arduino platform. A library is basically a class, so it should show you how its all done. On Arduino you can use classes, but there are a few restrictions: No new and delete keywords; No exceptions; No libstdc++, hence no standard functions, templates or classes
How do I write a Class in Arduino Language
Apr 26, 2008 · ...is a Library in C++ the same like a Class in Java? A library in the Arduino environment is typically written as a class but it does not need to be one. It just happens that the Arduino documentation on writing a class happens to be in a library tutorial. A C++ class is similar but not exactly the same as a java class.
Class and constructor. - Programming - Arduino Forum
May 5, 2020 · Each instance of the class "S" has a unique ID. Then there are two classes, Good and Bad, that have a member of type S. One uses an initializer list, the other does not. In this example, "Good" and "Bad" are like "blinker" in your code, and their member "S s" is like the member "byte LedPin" in your "blinker" class.
Declare functions and classes in sketch - Arduino Forum
Jun 26, 2014 · void myfunction ( myClass* arg ) ; // at this point, the details of class are not known, but the compiler knows there is a class // with that name, which can have a pointer to it. // more stuff // more stuff. class myClass {// actual class definition} You sometimes need to do this, if class A refers to class B and class B also refers to class A.
class - Using C++ classes in Arduino - Stack Overflow
May 9, 2016 · I need to use classes in Arduino to be able to multitask. I've found a class example in here but I wanted to keep my main code clean, so I've decided to put the class in .h and .cpp files. After a bit of googling this is what I've came up with: Work.h file:
How to use stream.read( ) function for I2C communication
May 9, 2021 · For example the Serial object uses the classes 'Stream' and 'Print' for Serial/UART communication. The C++ language can make a derived class from another class. The object can use the functions of both. The object is "Wire" and you can use "Wire.read()" and "Wire.readBytes()". The Stream class is the base class.
How do I use enum? - Programming - Arduino Forum
Aug 30, 2011 · Please read - Arduino Playground - Enum Resource - about how to use enum. and - scope - Arduino Reference - about variable scope system August 30, 2011, 8:49pm
[SOLVED] Creating instance of another class inside a class
Feb 4, 2019 · Dear everyone I've been building small Arduino projects as a hobby for a few years now. The devices are getting more complicated and I've ran into a situation, where my sketches get long with a bunch of variables and functions. Thus, it starts to make sense to create classes to make projects more manageable and also expandable (I might want to add a second or third led, maybe have an ...
c++ - Vectors in Arduino - Stack Overflow
In Arduino Uno (and other ATmega-based boards) an int stores a 16-bit (2 bytes) value. This guarantees a range from -32.768 to 32.767 (a minimum value of -2 ^ 15 and a maximum value of (2 ^ 15) - 1). In Arduino Due and other boards based on SAMD computers (such as MKR1000 and Zero), an int stores a 32-bit value (4 bytes).