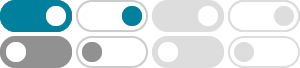
Take input from user and store in .txt file in Python
Nov 7, 2022 · In this article, we will see how to take input from users and store it in a .txt file in Python. To do this we will use python open () function to open any file and store data in the file, we put all the code in Python try-except block.
Take user input and put it into a file in Python? - Stack Overflow
Jun 10, 2010 · Solution for Python 3.1 and up: filename = input("filename: ") with open(filename, "w") as f: f.write(input()) This asks the user for a filename and opens it for writing. Then everything until the next return is written into that file. The "with... as" statement closes the file automatically.
Reading and Writing to text files in Python - GeeksforGeeks
Jan 2, 2025 · There are three ways to read txt file in Python: Reading From a File Using read () read (): Returns the read bytes in form of a string. Reads n bytes, if no n specified, reads the entire file. Reading a Text File Using readline () readline (): Reads a line of the file and returns in form of a string.For specified n, reads at most n bytes.
How to Write to Text File in Python
To write to a text file in Python, you follow these steps: First, open the text file for writing (or append) using the open() function. Second, write to the text file using the write() or writelines() method. Third, close the file using the close() method. The following shows the basic syntax of the open() function: f = open(file, mode)
Python File Write - W3Schools
To write to an existing file, you must add a parameter to the open() function: Open the file "demofile2.txt" and append content to the file: f.write ("Now the file has more content!") Open the file "demofile3.txt" and overwrite the content: f.write ("Woops! I have deleted the content!") Note: the "w" method will overwrite the entire file.
Writing to file in Python - GeeksforGeeks
Dec 19, 2024 · Writing to a file in Python means saving data generated by your program into a file on your system. This article will cover the how to write to files in Python in detail. Creating a file is the first step before writing data to it. In Python, we can create a …
Python Read And Write File: With Examples
Jun 26, 2022 · Learn how to open, read, and write files in Python. In addition, you'll learn how to move, copy, and delete files. With many code examples.
Python Write to Text File: A Comprehensive Guide - CodeRivers
Mar 21, 2025 · Whether you're logging data, saving configuration settings, or generating reports, writing to a text file allows you to store information persistently. This blog post will delve into the fundamental concepts, usage methods, common practices, and best practices of …
How to save user input to a File in Python | bobbyhadz
Apr 9, 2024 · To save user input to a file: Use the with open() statement to open the file in write mode. Use the input() function to take input from the user. Use the file.write() method to write the input to the file. The with statement automatically closes the file.
File Handling in Python – How to Create, Read, and Write to a File
Aug 26, 2022 · Below is the code required to create, write to, and read text files using the Python file handling methods or access modes. In Python, you use the open() function with one of the following options – "x" or "w" – to create a new file: