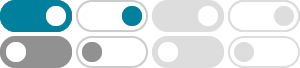
Binary Search Tree In Python - GeeksforGeeks
Feb 10, 2025 · A Binary search tree is a binary tree where the values of the left sub-tree are less than the root node and the values of the right sub-tree are greater than the value of the root node. In this article, we will discuss the binary search tree in …
Python Program For Binary Search Tree (Insert, Search ... - Python …
How to write a binary search tree program using Python? To write a binary search tree program in Python, you can start by defining a Node class to represent each node in the tree. Then, create a BinarySearchTree class to manage the operations on the tree, such as …
Python Object-Oriented Programming: Binary search tree class …
Mar 29, 2025 · Learn object-oriented programming (OOP) in Python by creating a class that represents a binary search tree. Implement methods for inserting elements into the tree and searching for specific values.
Binary Search Tree Implementation in Python - AskPython
Feb 12, 2021 · To implement a Binary Search Tree, we will use the same node structure as that of a binary tree which is as follows. def __init__(self, data): self.data = data. self.leftChild = None. self.rightChild=None.
Binary Search Tree in Python - PythonForBeginners.com
Sep 1, 2021 · How to search an element in a Binary search Tree? As you know that a binary search tree cannot have duplicate elements, we can search any element in a binary search tree using the following rules that are based on the properties of the binary search trees. We will start from the root and follow these properties
python - How to implement a binary tree? - Stack Overflow
Apr 8, 2010 · Here is my simple recursive implementation of binary search tree. def __init__(self, val): self.l = None. self.r = None. self.v = val. def __init__(self): self.root = None. def get_root(self): return self.root. def add(self, val): if not self.root: self.root = Node(val) else: self._add(val, self.root) def _add(self, val, node): if val < node.v:
How to implement a binary search tree in Python?
A (simple idea of) binary tree search would be quite likely be implement in python according the lines: def search(node, key): if node is None: return None # key not found if key< node.key: return search(node.left, key) elif key> node.key: return search(node.right, …
Writing a Binary Search Tree in Python with Examples - Boot.dev …
Oct 1, 2022 · A binary search tree, or BST for short, is a tree where each node is a value greater than all of its left child nodes and less than all of its right child nodes. Read on for an implementation of a binary search tree in Python from scratch!
Python: Binary Search Tree (BST)- Exercises, Practice, Solution
Apr 1, 2025 · Write a Python program to check whether a given binary tree is a valid binary search tree (BST) or not. Let a binary search tree (BST) is defined as follows: The left subtree of a node contains only nodes with keys less than the node's key.
How to implement Binary Search Tree in Python [Easy Examples]
Mar 14, 2022 · The knowledge of how to implement binary search tree in Python is very useful while working on real time applications. In many situations, we will need to perform the operations on binary search tree in Python. In this tutorial, we covered creation, insertion, deletion and traversal on binary search tree with the sample code example.
- Some results have been removed