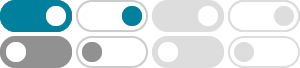
Java Program for Merge Sort - GeeksforGeeks
Oct 23, 2024 · Merge Sort is a comparison-based sorting algorithm that uses divide and conquer paradigm to sort the given dataset. It divides the dataset into two halves, calls itself for these two halves, and then it merges the two sorted halves.
Merge Sort in Java - Baeldung
Jul 25, 2024 · For the implementation, we’ll write a mergeSort function that takes in the input array and its length as the parameters. This will be a recursive function, so we need the base and the recursive conditions.
java - Merge Sort Recursion - Stack Overflow
This is a code from Introduction to Java Programming about Merge Sort. This method uses a recursion implementation. public class MergeSort { 2 /** The method for sorting the numbers */ 3
Merge Sort in Java: Algorithm & Implementation (with code)
May 25, 2023 · Here is the simple algorithm for Merge Sort implementation using recursion: We create a recursive function say mergeSort () that takes arr to be sorted as input and the first and last index of the array. Initially, we pass the first index as 0 and the last as the length of array-1.
Merge Sort – Algorithm, Implementation and Performance
Mar 4, 2023 · Merge sort functions by partitioning the input into smaller sub-arrays, sorting each sub-array recursively, and subsequently merging the sorted sub-arrays.
Merge Sort with Java - Java Challengers
May 8, 2023 · The merge sort is a sorting algorithm that uses a divide-and-conquer strategy to sort an array of elements. It is an efficient sorting algorithm, with an average time complexity of O(n log n). The merge sort algorithm works by recursively dividing the input array into two halves until each half contains only one element or is empty.
Java recursion and Merge Sort - Stack Overflow
May 12, 2014 · int [] b = mergeSub(array, mid+1, right); return merge(a, b); int[] arr=new int[1]; arr[0]=arr[left]; return arr; int index =0; int indexLeft =0; int indexRight=0; int[] result = new int[left.length+right.length]; while(indexLeft<left.length && indexRight<right.length){ if(left[indexLeft] <= right[indexRight]) result[index]=left[indexLeft];
sorting - Recursive Merge Sort Java Program - Stack Overflow
Mar 26, 2013 · public static int[] mergesort(int[] data, int low, int high) int middle = (high+low)/2; if (middle==low) int[] data2 = new int [1]; data2[0]=data[middle]; return data2; else. int[] firstHalfSorted = mergesort(data, low, middle); int[] secondHalfSorted = mergesort(data, middle+1, high); return (merge(firstHalfSorted, secondHalfSorted));
Merge Sort with Recursion: A Comprehensive Guide
Jan 12, 2025 · How Merge Sort works with recursion. Recursion tree visualization for deeper understanding. Implementation for both arrays and linked lists in Java.
Merge Sort using recursion | MyCareerwise
Merge sort is a comparison-based sorting algorithm that follows a divide and conquers paradigm to sort the elements in ascending or descending order. Though it is a comparison based sorting technique, it is different from bubble or selection sort.