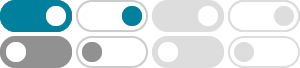
Python Program to Find the Factorial of a Number
The factorial of a number is the product of all the integers from 1 to that number. For example, the factorial of 6 is 1*2*3*4*5*6 = 720. Factorial is not defined for negative numbers, and the factorial of zero is one, 0! = 1. Factorial of a Number using Loop # Python program to find the factorial of a number provided by the user.
Factorial of a Number – Python | GeeksforGeeks
Apr 8, 2025 · This code calculates the factorial of a number by first finding the prime factors of each number from 2 to n, and then multiplying them together to compute the factorial. The prime factors are stored along with their powers for efficient multiplication.
factorial() in Python - GeeksforGeeks
Jul 9, 2024 · Not many people know, but python offers a direct function that can compute the factorial of a number without writing the whole code for computing factorial. Naive method to compute factorial. Using math.factorial () This method is defined in “math” module of python. Because it has C type internal implementation, it is fast.
Python program to find the factorial of a number using recursion
Jan 31, 2023 · In this article, we are going to calculate the factorial of a number using recursion. Examples: Output: 120. Input: 6. Output: 720. Implementation: If fact (5) is called, it will call fact (4), fact (3), fact (2) and fact (1). So it means keeps calling itself by …
Factorial of a Number in Python - Python Guides
Mar 20, 2025 · This Python tutorial explains, how to print factorial of a number in Python, Python program to print factorial of a number using function, Python program to find factorial of a number using while loop, etc.
Function for factorial in Python - Stack Overflow
Jan 6, 2022 · The easiest way is to use math.factorial (available in Python 2.6 and above): If you want/have to write it yourself, you can use an iterative approach: fact = 1. for num in range(2, n + 1): fact *= num. return fact. or a recursive approach: if n < 2: return 1. else: return n * factorial(n-1)
Write a Python Program to Find the Factorial of a Number
Feb 5, 2025 · Learn how to find the factorial of a number in Python using loops, recursion, and the math module. The factorial of a number is the product of all positive integers from 1 to that number.
Python Program For Factorial (3 Methods With Code) - Python …
To write a factorial program in Python, you can define a function that uses recursion or iteration to calculate the factorial of a number. Here is an example using recursion: def factorial(n): if n == 0: return 1 else: return n * factorial(n-1)
Factorial Program in Python(Factorial of a Number in Python)
Oct 19, 2024 · In Python, calculating the factorial of a number can be done in several ways: using loops, recursion, or built-in functions. In this article, we will explore various ways to write a program to find the factorial of a number in Python, covering loops and recursion. What is the Factorial of a Number?
Factorial of a number using userdefined function in Python
In this tutorial, we will learn how to find the factorial of a given number without using the inbuilt function i.e math.factorial () in Python. Python providing a fantastic set of libraries which are very useful and makes the work much easier, But here is the catch, we will learn to …
- Some results have been removed