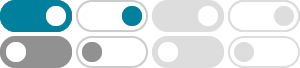
Python: self.__class__ vs. type(self) - Stack Overflow
Apr 25, 2017 · I would use self.__class__ since it's more obvious what it is. However, type(t) won't work for old-style classes since the type of an instance of an old-style class is instance while the type of a new-style class instance is its class: >>> class Test(): pass >>> t = Test() >>> type(t) is t.__class__ False >>> type(t) instance
python - What is the purpose of the `self` parameter? Why is it …
def func(self, name): self.name = name. I know that self refers to the specific instance of MyClass. But why must func explicitly include self as a parameter? Why do we need to use self in the method's code? Some other languages make this implicit, or use special syntax instead.
Python's Self Type: How to Annotate Methods That Return self
In this tutorial, you'll learn how to use the Self type hint in Python to annotate methods that return an instance of their own class. You'll gain hands-on experience with type hints and annotations of methods that return an instance of their class, making your code more readable and maintainable.
Self Type in Python - GeeksforGeeks
Apr 19, 2023 · Self-type is a brand-new function added to Python 3.11. A method or function that returns an instance of the class it belongs to is defined by the self type. In situations where we wish to enforce that a method returns an instance of …
self in Python class - GeeksforGeeks
Feb 26, 2025 · What is the Purpose of “self”? In Python, self is used as the first parameter in instance methods to refer to the current object. It allows methods within the class to access and modify the object’s attributes, making each object independent of others.
Python Self - W3Schools
The self Parameter. The self parameter is a reference to the current instance of the class, and is used to access variables that belongs to the class. It does not have to be named self, you can call it whatever you like, but it has to be the first parameter of any function in the class:
Python: self vs type(self) and the proper use of class variables
Jul 24, 2014 · When using a class variable in Python, one can access and (if it's mutable) directly manipulate it via "self" (thanks to references) or "type (self)" (directly), while immutable variables (e.g., integers) apparently get shadowed by new instance objects when you just use "self".
Self Type in Python: Simplifying Object-Oriented Programming
Sep 28, 2023 · In this article, we’ll delve into the intriguing world of the “Self” type in Python. We will explore what it is, how it works, and how it can simplify your OOP endeavors.
Understanding Python self Variable with Examples - AskPython
Sep 5, 2019 · Python self variable is used to bind the instance of the class to the instance method. We have to explicitly declare it as the first method argument to access the instance variables and methods. This variable is used only with the instance methods.
Python's Self Type | Annotate Method That Return Self Type
Aug 29, 2024 · Starting from Python version 3.11 and beyond, the Self type, as defined by PEP 673, is the recommended annotation for methods that return an instance of their class. The Self type can be directly imported from the `typing` module. However, for Python versions earlier than 3.11, the Self type is available in the `typing_extensions` module.