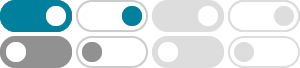
java - Creating a triangle with for loops - Stack Overflow
I need to draw a simple triangle using for loops. I can make a half triangle, but I don't know how to add to my current loop to form a full triangle. for (int i = 0; i < 6; i++) { for (int j = 0; j < i; j++) { System.out.print("*"); } System.out.println(""); }
Printing Triangle Pattern in Java - GeeksforGeeks
Mar 13, 2023 · The first loop within the outer loop is used to print the spaces before each star. As you can see the number of spaces decreases with each row while we move towards the base of the triangle, so this loop runs one time less with each iteration.
Java Pattern Programs – Learn How to Print Pattern in Java
Apr 8, 2025 · Here, you will find the top 25 Java pattern programs with their proper code and explanation. 1. Square Hollow Pattern. 2. Number Triangle Pattern. 3. Number-Increasing Pyramid Pattern. 4. Number-Increasing Reverse Pyramid Pattern. 5. Number-Changing Pyramid Pattern. 6. Zero-One Triangle Pattern. 7. Palindrome Triangle Pattern. 8. Rhombus Pattern.
Creating a Triangle with for Loops in Java - Baeldung
Jan 25, 2024 · First, we’ve studied the right triangle, which is the simplest type of triangle we can print in Java. Then, we’ve explored two ways of building an isosceles triangle. The first one uses only for loops and the other one takes advantage of the StringUtils.repeat() and the String.substring() method and helps us write less code.
Triangle Word Pattern using Nested Loops in Java
This should be simple, as you've already figured out the middle of the triangle and all you need to do is make the loop shorter based on which character is being printed (similar to how you're already deciding which character to print). That'll get you the left side.
Java For Loop triangle pattern - Stack Overflow
for(int i = n; i>=1; i--){ //This is the same loop, but written in a different way. for(int j = 0; i < n-i; j++){ System.out.print(" "); //THREE SPACES HERE } for(int k = 1; k<=i; k++){ System.out.print(x+" "); x+=5; } System.out.println(); }
Java program to display triangle alphabet pattern
Aug 16, 2024 · In this tutorial, we will discuss a concept of Java program to display triangle alphabet pattern using for loop in java language. here, we displayed 15 alphabet Floyd’s triangle program with coding and using nested for loop and also …
Java program to Integrated triangle patterns using for loop
Aug 15, 2024 · In this tutorial, we will discuss a concept of Java program to Integrated triangle patterns using for loop in Java language. In Java programming language, we can use for loop ,while loop and do-while loop to display different number (binary, decimal), alphabets or star patterns programs.
Mastering Java: How to Print Triangles Using Nested Loops
In this tutorial, we will explore how to print various triangle patterns in Java using nested loops. Understanding how to manipulate loops is foundational for programming, and printing shapes is a fun way to practice your skills.
How to print a triangle rectangle pattern (from left to right and …
Sep 20, 2019 · In this brief article, we will explain you easily a way of drawing the famous triangles using loops in Java. As logic to print a left oriented triangle with asterisks in Java, we will create a loop that will iterate the number of rows that the user wants for the triangle.