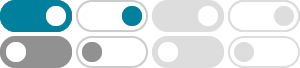
Reverse a String in Java - GeeksforGeeks
Mar 27, 2025 · In this article, we will discuss multiple approaches to reverse a string in Java with examples, their advantages, and when to use them. The for loop is a simple, straightforward approach to reverse a string in Java that offers full control over the reversal process without relying on additional classes.
java - How to make a reverse string using a for loop ... - Stack Overflow
Apr 1, 2017 · public String reverseString(String str) { String res = ""; for (int i = 0; i < str.length(); i++) { res = str.charAt(i) + res; // Add each char to the *front* } return res; } Note also the simpler, canonical, loop termination condition.
java - Using loops to reverse a string - Stack Overflow
Mar 10, 2021 · To simplify the code, you can use an enhanced for loop instead of reversed for loop and swap the summands inside: String str = "hello world", reverse = ""; for (char ch : str.toCharArray()) reverse = ch + reverse; System.out.println(reverse); // dlrow olleh
Java How To Reverse a String - W3Schools
You can easily reverse a string by characters with the following example: reversedStr = originalStr.charAt(i) + reversedStr; } System.out.println("Reversed string: "+ reversedStr);
Reverse a string in Java - Stack Overflow
Sep 10, 2017 · StringBuffer buffer = new StringBuffer(str); System.out.println("StringBuffer - reverse : "+ buffer.reverse() ); String builderString = (new StringBuilder(str)).reverse().toString; System.out.println("StringBuilder generated reverse String : "+ builderString );
Reverse a String Using a For Loop in Java - Tpoint Tech
Sep 10, 2024 · Reversing a string is a common project in programming, and it can be accomplished the use of diverse strategies. One such technique is with the aid of the use of a for loop in Java. In this text, we are able to discover how to reverse a string using a for loop and provide example program.
How To Reverse A String In Java – Learn 5 Easy Methods
May 30, 2021 · You can reverse a string using java with the below approaches. We are given below 5 methods from simplest one to advanced one which you can use to reverse a string according to your requirement. Approaches to reverse a String: Reverse a String in Java using for loop; Java program to reverse a String using while loop
How to Reverse a String in Java Using for Loop - Hero Vired
Mar 19, 2024 · The toCharArray() method to reverse a string in Java uses the total length of the string variable. The for loop iterates up to the end of the string index zero. This approach to reverse a string in Java can employ the in-built reverse() method to receive your intended output.
String Reverse in Java Program | For | Recursion | Function ...
Jan 14, 2023 · In this article, you will learn how to reverse in Java program using for loop, recursion, and function. Enter a string to make reverse:: You should have knowledge of the following topics in Java programming to understand this program: public class Main { // It's the driver function public static void main(String[] args) {
How to Reverse a String in Java - Selenium Webdriver Appium …
Nov 4, 2019 · In this blog, we will learn how to reverse a string in Java. This will be asked in the most of the interviews. This can be implemented in four different ways listed below: Convert String into Character Array and use For loop; For loop with CharAt() method; Using StringBuffer class; Using StringBuilder class