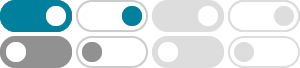
javascript - Remove falsy values in object - Stack Overflow
Feb 24, 2016 · for (var i in obj) { if (obj[i] === null || obj[i] === undefined) { delete obj[i]; } return obj; This is the actual question: Make a function that takes in an object, loops through all its properties, and removes any that are falsy. Then return the object that was passed in. (hint: delete)
javascript - Remove false values in object - Stack Overflow
Jun 1, 2015 · To remove those properties you can use this algorithm: for (k in myObj) { if (myObj.hasOwnProperty(k) && myObj[k] === false) { delete myObj[k]; } } If you are just interested in the keys of non-false values, you can use: var keys = Object.keys(myObj).filter(function (k) { return myObj[k] !== false; });
Is there a nice way in javascript to removing Falsy values from a ...
Feb 7, 2018 · The answer to "can I do x to an object" (or an array for that matter) is usually "yes" and it frequently involves some form of reduce. If you want to filter falsy values you could do something like this:
How to remove the falsy value attribute from object
Apr 8, 2021 · Sometimes we want to remove null, empty, undefined, false value attribute from an object. we can do this using the below method. const removeFalsyElement = object => { const newObject = {}; Object.keys(object).forEach(key => { if (object[key]) { newObject[key] = object[key]; } }); return newObject; };
JavaScript: Remove all falsy values from an object or array
Mar 3, 2025 · Write a JavaScript program to remove all false values from an object or array. Use recursion. Initialize the iterable data, using Array.isArray(), Array.prototype.filter() and Boolean for arrays in order to avoid sparse arrays.
How to remove falsy values from an array in JavaScript
Jun 5, 2024 · Using Array.prototype.flatMap(), you can remove falsy values by mapping each element to an array containing the element if it’s truthy, or an empty array if it’s falsy. The resulting arrays are then flattened into a single array of truthy values.
Recursively Remove Falsy Values | JavaScript Tutorials - LabEx
To deeply remove all falsy values from an object or array, use the following algorithm: Use recursion to call the compactObject() function on each nested object or array. Initialize the iterable data using Array.isArray() , Array.prototype.filter() , and Boolean() .
Remove Falsy values (or empty strings) from JavaScript objects
May 10, 2016 · This method iterates over an object and removes all keys with falsy values. That is, falsy values will cause the key/value pair to be removed from the object. This is very useful for removing unwanted data from an object.
Remove False Properties in JavaScript - Online Tutorials Library
Oct 12, 2020 · Learn how to iterate over an object in JavaScript and remove properties with false values. Step-by-step guide with examples.
javascript - How can I delete falsy elements in an Object
Jul 1, 2015 · When you use for-in, the iteration variable is set to the property name, not the value (it's not like PHP foreach). You need to use user[prop] to get the value. Also, you should return after the entire loop -- you're returning as soon as you find a truthy property.