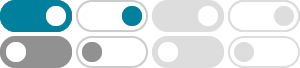
Binary Search (Recursive and Iterative) - Python - GeeksforGeeks
Feb 21, 2025 · Binary Search Algorithm is a searching algorithm used in a sorted array by repeatedly dividing the search interval in half. The idea of binary search is to use the information that the array is sorted and reduce the time complexity to O (log N). Below is the step-by-step algorithm for Binary Search:
Binary Search in Python
Binary search is a powerful algorithm that allows us to find a target value in a sorted list of items quickly and efficiently. By dividing the search space in half repeatedly, we can drastically reduce the number of comparisons we need to make, making it much faster than a linear search.
Python Program For Binary Search (With Code) - Python Mania
In this article, we will delve into the intricacies of the binary search algorithm and provide a comprehensive guide on how to implement a Python program for binary search. What is Binary Search? Binary search is a search algorithm that finds the position of a target value within a sorted collection of elements.
Binary Search in Python – How to Code the Algorithm with …
Jul 18, 2022 · Binary search algorithms are also known as half interval search. They return the position of a target value in a sorted list. These algorithms use the “divide and conquer” technique to find the value's position.
Binary Search in Python: A Guide for Efficient Searching
Aug 23, 2024 · Learn how to implement binary search in Python using iterative and recursive approaches, and explore the built-in bisect module for efficient, pre-implemented binary search functions.
How to Do a Binary Search in Python
Watch it together with the written tutorial to deepen your understanding: Creating a Binary Search in Python. Binary search is a classic algorithm in computer science. It often comes up in programming contests and technical interviews. Implementing binary search turns out to be a challenging task, even when you understand the concept.
Binary Search in Python (Recursive and Iterative)
Learn what is Binary Search Algorithm. Create Project for Binary Search Algorithm using Python modules like Tkinter for GUI.
Binary Search in Python - Python Guides
Mar 20, 2025 · In this tutorial, I explained binary search in Python. I discussed methods like implementing binary search iteratively, implementing binary search recursively, and using Python’s built-in functions. I also covered use cases, advanced binary search techniques, and optimizing binary search in Python. You may read:
Python Program for Binary Search in Python - Studytonight
Aug 12, 2021 · In this tutorial, we will perform a binary search operation to discover an element's index position in a list with two different methods. Binary search is the most popular program for searching. Let's say we have a thousand-element list and …
Python Program for Binary Search (Recursive and Iterative)
Oct 6, 2019 · In this blog post, we will explore both recursive and iterative implementations of the binary search algorithm in Python. Additionally, we’ll provide detailed explanations of the logic behind each approach and showcase program outputs.