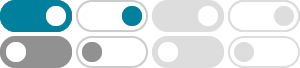
How to declare and add items to an array in Python
Arrays (called list in python) use the [] notation. {} is for dict (also called hash tables, associated arrays, etc in other languages) so you won't have 'append' for a dict. If you actually want an array (list), use: array = [] array.append(valueToBeInserted)
How to Add Element to Array in Python - GeeksforGeeks
Nov 29, 2024 · The simplest and most commonly used method to append an element to an array in Python is by using append() method. It’s straightforward and works in-place, meaning it modifies the original array directly.
How to append an Array in Python? - AskPython
Jun 30, 2020 · Python append() function enables us to add an element or an array to the end of another array. That is, the specified element gets appended to the end of the input array. The append () function has a different structure according …
Python appending array to an array - Stack Overflow
Oct 31, 2016 · If you want to append the lists and keep them as lists, then try: result = [] result.append(a) result.append(b) print result # [[1, 2, 3], [10, 20]]
How to Append to an Array in Python? - Python Guides
Dec 28, 2024 · Learn how to append elements to an array (or list) in Python using methods like `append()`, `extend()`, and NumPy's `np.append()`. Step-by-step examples included
Python Array Add: How to Append, Extend & Insert Elements
Apr 15, 2025 · Learn how to add elements to an array in Python using append(), extend(), insert(), and NumPy functions. Compare performance and avoid common errors.
Python Arrays - W3Schools
You can use the append() method to add an element to an array. Add one more element to the cars array: You can use the pop() method to remove an element from the array. Delete the second element of the cars array: You can also use the remove() method to remove an element from the array. Delete the element that has the value "Volvo":
Python Array Append: A Comprehensive Guide - CodeRivers
Jan 23, 2025 · One of the most basic yet essential operations is appending elements to an array. Appending allows you to add new elements to the end of an existing array, expanding its size and content. This blog post will dive deep into the concept of Python array append, exploring its usage methods, common practices, and best practices.
Python Add Array Item - GeeksforGeeks
Dec 8, 2024 · If we're working with arrays in Python, we might often need to add items to an array. In this article, we’ll explore how to add items to an array using different methods. Add single element - Using append() The append() method adds a single element to the end of the array.
Mastering `array.append` in Python - CodeRivers
3 days ago · In Python, the `array` module provides an `array` data type which is more space-efficient than the built-in `list` for storing homogeneous data (data of the same type). The `append` method in the `array` class is a crucial operation that allows you to add new elements to the end of an existing `array`. Understanding how to use `array.append` effectively can …