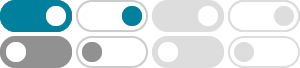
Binary Tree in Python - GeeksforGeeks
Feb 27, 2025 · The topmost node in a binary tree is called the root, and the bottom-most nodes are called leaves. Introduction to Binary Tree Representation of Binary Tree. Each node in a Binary Tree has three parts: Data; Pointer to the left child; Pointer to the right child; Binary Tree Representation Create/Declare a Node of a Binary Tree in Python
Trees in Python - GeeksforGeeks
Mar 4, 2025 · 1. Binary Tree. Binary Tree is a non-linear and hierarchical data structure where each node has at most two children referred to as the left child and the right child. The topmost node in a binary tree is called the root and the bottom-most nodes are called leaves. Explore in detail about Binary Tree in Python. 2. Binary Search Tree
python - How to implement a binary tree? - Stack Overflow
Apr 8, 2010 · A Tree is an even more general case of a Binary Tree where each node can have an arbitrary number of children. Typically, each node has a 'children' element which is of type list/array. Now, to answer the OP's question, I am including …
Python Binary Tree - Online Tutorials Library
Learn about Python binary trees, their properties, types, and implementation details. Explore how to create and manipulate binary tree structures in Python.
Binarytree Module in Python - GeeksforGeeks
Jan 10, 2023 · The binary search tree is a special type of tree data structure whose inorder gives a sorted list of nodes or vertices. In Python, we can directly create a BST object using binarytree module. bst() generates a random binary search tree and return its root node.
Tree Data Structure in Python - PythonForBeginners.com
Jun 9, 2023 · We can define a Tree node of the structure shown above in Python using classes as follows. Here, the constructor of the Tree node takes the data value as input, creates an object of BinaryTreeNode type and initializes the data field equal to the given input, and initializes the references to the children to None.
Binary Trees in Python: A Comprehensive Guide - CodeRivers
Mar 17, 2025 · A binary tree is a tree - like data structure where each node has at most two children. Each node in a binary tree consists of a value and references (or pointers in languages like C/C++) to its left and right child nodes. Types of Binary Trees. Full Binary Tree: Every node has either 0 or 2 children.
Binary Trees in Python: Implementation and Examples
Jun 19, 2024 · Nodes: In a binary tree, a node represents a single element of the tree. Each node contains a value or data, and pointers (or references) to its left and right children, if they exist. Edges: Edges are the connections between nodes. An edge connects a parent node to a child node. Root: The root is the topmost node in a binary tree.
How can I implement a tree in Python? - Stack Overflow
Mar 1, 2010 · For example, a binary tree might be: def __init__(self): self.left = None. self.right = None. self.data = None. You can use it like this: If you need an arbitrary number of children per node, then use a list of children: def __init__(self, data): self.children = [] self.data = data.
Exploring Binary Trees in Python: Concepts, Usage, and Best …
Feb 10, 2025 · In Python, we can represent a binary tree node as a class. Each node will have a value, a reference to its left child, and a reference to its right child. def __init__(self, value): self.value = value. self.left = None. self.right = None. We can create a simple binary tree by instantiating multiple TreeNode objects and connecting them.
- Some results have been removed