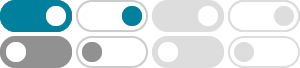
How do I multiply each element in a list by a number?
Feb 3, 2016 · A blazingly faster approach is to do the multiplication in a vectorized manner instead of looping over the list. Numpy has already provided a very simply and handy way for this that you can use. >>> import numpy as np >>> >>> my_list = np.array([1, 2, 3, 4, 5]) >>> >>> my_list * 5 array([ 5, 10, 15, 20, 25])
Python – Constant Multiplication over List - GeeksforGeeks
Feb 1, 2025 · Using Numpy we can perform element-wise multiplication on entire array by multiplying Numpy array by constant. This allows for efficient and fast operations resulting in modified array. Explanation: List a is converted into a NumPy array which allows for efficient element-wise operations.
python - How to multiply individual elements of a list with a number …
If you use numpy.multiply. S = [22, 33, 45.6, 21.6, 51.8] P = 2.45 multiply(S, P) It gives you as a result. array([53.9 , 80.85, 111.72, 52.92, 126.91])
python - Numpy, multiply array with scalar - Stack Overflow
Nov 26, 2018 · You can multiply numpy arrays by scalars and it just works. >>> import numpy as np >>> np.array([1, 2, 3]) * 2 array([2, 4, 6]) >>> np.array([[1, 2, 3], [4, 5, 6]]) * 2 array([[ 2, 4, 6], [ 8, 10, 12]])
Multiplying a Constant to All Elements in a NumPy Array - Python …
Discover how to multiply a constant to each element of a NumPy array using the multiplication operator. This tutorial provides clear examples and step-by-step instructions for working with NumPy arrays.
numpy.multiply — NumPy v2.2 Manual
numpy.multiply# numpy. multiply (x1, x2, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None, subok=True [, signature]) = <ufunc 'multiply'> # Multiply arguments element-wise. Parameters: x1, x2 array_like. Input arrays to be multiplied. If x1.shape!= x2.shape, they must be broadcastable to a common shape (which becomes the ...
numpy.multiply() in Python - GeeksforGeeks
5 days ago · By mastering numpy.multiply() we can efficiently handle element-wise multiplication across arrays and scalars.
Multiplying an array by a constant - University of Utah
When we multiply an array by a constant, each element is multiplied by that constant. >>> print (2*M) [ [ 2. -4. 2.] [ 4. -8. 4.] [ 6. -12. 6.] [ 8. -16. 8.] [ 10. -20. 10.]]
Multiply Every Element in a Numpy Array: A Guide
Jul 23, 2023 · One of the most common operations in data science is element-wise multiplication, where each element in an array is multiplied by a certain value. This can be done easily in Numpy using the * operator or the np.multiply() function.
Python: Multiply Lists (6 Different Ways) - datagy
Dec 12, 2021 · In this tutorial, you learned two different methods to multiply Python lists: multiplying lists by a number and multiplying lists element-wise. You learned how to simplify this process using numpy and how to use list comprehensions and Python for loops to multiply lists.