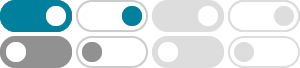
How to find maximum between two numbers in javascript using switch case ...
Aug 11, 2018 · const numOne = 5; const numTwo = 10; console.log(`Bigger number is: ${Math.max(numOne, numTwo)}`); Or if you absolutely 'have to' use switch statement , you can try something like this:
Maximum of Two Numbers in JavaScript - GeeksforGeeks
Feb 21, 2024 · Example: Finding maximum of two numbers using the conditional operator. This approach utilizes the Math.max () method along with the spread syntax (...) to find the maximum of two numbers.
Program to Find the Largest Number using Ternary Operator
Jun 24, 2022 · The task is to write a program to find the largest number using ternary operator among: Two Numbers; Three Numbers; Four Numbers; Examples: Input : 10, 20 Output : Largest number between two numbers (10, 20) is: 20 Input : 25 75 55 15 Output : Largest number among four numbers (25, 75, 55, 15) is: 75. A Ternary Operator has the following form,
JavaScript: Accept two integers and display the larger - w3resource
Feb 28, 2025 · Write a JavaScript function that compares two integers by converting them to binary strings and determining the larger one. Write a JavaScript function that finds the largest of two integers using arithmetic operations to simulate conditional logic.
How to find largest of three numbers using JavaScript
Jan 3, 2024 · Below are the approaches to finding the largest of three numbers using JavaScript: This is a straightforward approach using if-else statements to compare the numbers and find the largest one. Example: In this example, we are using a Conditional Statement (if-else). The Math.max() method can be used to find the maximum of a list of numbers.
conditional statements - Write a JavaScript program that accept two ...
Jul 3, 2018 · function integer(a,b) { var isInteger = Number.isInteger(a) && Number.isInteger(b); if(isInteger && a === b) document.write("Both numbers are equal!"); else if(isInteger) document.write("The larger number is " + Math.max(a,b)); else document.write("Please add an integer!"); } integer(-1,-1);
Mastering JavaScript – Finding the Maximum of Two Numbers …
JavaScript provides a built-in method, Math.max(), that can be used to find the maximum of multiple numbers, including two numbers. This method eliminates the need for manual comparisons or conditional statements, simplifying the process of finding the maximum.
Find the larger of two numbers in JavaScript - Includehelp.com
Oct 22, 2017 · We will create a function to return the larger of two numbers when given numbers as parameters to them. The basic logic behind this program is that we check if one is greater than other, than simply return the first one otherwise, of course another number will be greater.
conditional operator - JavaScript 'if' alternative - Stack Overflow
Aug 9, 2012 · For example, it is easy to get the minimum or maximum of two numbers using a ternary operator, but it becomes clumsy to find the largest and second largest among three or more numbers and is not even recommended. It is better to use if..else instead.
How to Find a Larger Number of Two Numbers in JavaScript
May 8, 2023 · By developing these skills, you will quickly learn how to write a JavaScript program to get the maximum of two numbers using any of the several methods available. 1. Using JavaScript Math.max () Method. 2. Using JavaScript Conditional Statement. 3. Using JavaScript Ternary Operator. 4. Using JavaScript Sort () Method. 1.
- Some results have been removed