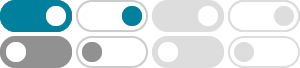
javascript - Remove characters from a string - Stack Overflow
You may remove a single occurrence of a character from a string with something like the following: const removeChar = (str: string, charToBeRemoved: string) => { const charIndex: number = str.indexOf(charToBeRemoved); let part1 = str.slice(0, charIdx); let part2 = str.slice(charIdx + 1, str.length); return part1 + part2; };
How can I remove a character from a string using JavaScript?
You can make the following function to remove characters at start index to the end of string, just like the c# method first overload String.Remove(int startIndex): function Remove(str, startIndex) { return str.substr(0, startIndex); }
Remove a Character From String in JavaScript - GeeksforGeeks
Nov 17, 2024 · This method is used to remove all occurrences of a specified character or string from the input string, unlike the previous method, which removes only the first occurrence. It uses a regular expression with the global property to select and remove every occurrence.
JS Remove Char from String – How to Trim a Character from a String …
May 9, 2024 · To remove a specific character from a string in JavaScript, you can use the replace() method. Here is the general syntax for the replace() method: string.replace(pattern, replacement);
JavaScript – Remove Text From a String - GeeksforGeeks
Nov 16, 2024 · Here are the different approaches to remove specific text from a string in JavaScript, starting from the most commonly used approaches. The replace () method is a direct and widely used method to remove specific text by replacing it with an empty string “”.
How to remove characters from a string in JavaScript - Educative
JavaScript provides several built-in methods for removing characters from strings, each with unique functionalities: replace(): Replaces specified characters with a new string or removes them when an empty string is used. To replace all occurrences, combine it with a regular expression and the global flag.
11 Ways to Remove Character from String in JavaScript
Apr 25, 2020 · Here are the 11 Effective Ways to Remove Characters from Strings using JavaScript. The JavaScript substring () method retrieves characters between two indexes, returning a new substring. By setting startindex and endindex, you …
JavaScript - Delete Character at a Given Position in a String
Nov 21, 2024 · These are the following ways to Delete Character at a Given Position in a String: 1. Using slice () Method. The slice () method allows you to extract portions of a string. By slicing before and after the target position, you can omit the desired character. 2. …
Remove specific characters from a string in Javascript
If you wish to remove all occurrences of F0 from a given string, you can use the replaceAll() method. Another way to do it: It splits the string into two: ["", "123456"], then selects the last element. Regexp solution: plain solution: ref = ref.substr(2);
Remove a Substring from a String in JavaScript | by ryan - Medium
Sep 16, 2024 · You can remove a substring from a string in JavaScript using methods like replace(), regular expressions, split() and join(), indexOf() and substring(), or by creating a custom helper...