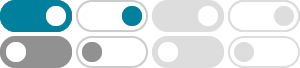
JavaScript: Dynamically Creating Variables for Loops
I was pretty proud of the way I made iterating variables with my code, was going to share it but I think I'll just sort of show you modified version of it. function variableBuilder() { let i = 0; while (i <= 20) { let accountVariable = "account".concat(i); `// variable can even be reassigned here` console.log(accountVariable); i++; } }
Is var necessary when declaring Javascript variables?
Oct 8, 2010 · For the unenlightened like myself, JavaScript basically has two scopes for variables: global and local. Variables assigned outside of a function are global, and variables assigned inside of a function, using the var keyword, are local (not rocket surgery).
Defining JavaScript variables inside if-statements
Because JavaScript's variables have function-level scope, your first example is effectively redeclaring your variable, which may explain why it is getting highlighted. On old versions of Firefox, its strict JavaScript mode used to warn about this redeclaration, however one of its developers complained that it cramped his style so the warning ...
javascript - What's the best way to initialize empty variables in JS ...
Jul 15, 2013 · If I know the variable will be object later, I use: var obj; but it doesn't really matter if I initialize it as null or undefined: var obj = null; or var obj = undefined; For strings I personall...
Global variables in Javascript across multiple files
May 29, 2010 · This code automatically combines files 1.js, 2.js, and 3.js into one master JavaScript file javascript.js, so there is just one global scope. Share Follow
Why would a JavaScript variable start with a dollar sign?
The reason I sometimes use php name-conventions with javascript variables: When doing input validation, I want to run the exact same algorithms both client-side, and server-side. I really want the two side of code to look as similar as possible, to simplify maintenance.
Javascript How to define multiple variables on a single line?
Apr 21, 2018 · Reading documentation online, I'm getting confused how to properly define multiple JavaScript variables on a single line. If I want to condense the following code, what's the proper JavaScript "strict" way to define multiple javascript variables on a single line? var a = 0; var b = 0; Is it: var a = b = 0; or. var a = var b = 0; etc...
javascript - Is defining every variable at the top always the best ...
May 15, 2015 · Just because all the variables are defined in one place, doesn't make it "organized" or more "readable". It's definitely more understandable to declare variables where they are used. That way, the reader can say "Okay, now this is the code for the Indexing Table parser.". It's neat and tidy, no conglomerations of variable soup with no context. –
performance - JavaScript variables declare outside or inside loop ...
Apr 12, 2015 · When you declare a variable inside a loop isn't it declaring as many times the loop runs? Obviously it will slap you when you are in 'use strict' mode. People have disagreed with Crockford without thinking about the original question. So it is always good to declare variables on top -- 1. For readability, 2. Making good habits.
Define a global variable in a JavaScript function - Stack Overflow
Global variables are declared outside of a function for accessibility throughout the program, while local variables are stored within a function using var for use only within that function’s scope. If you declare a variable without using var, even if it’s inside a function, it will still be seen as global.