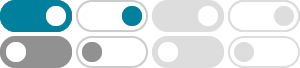
Loop through an array in JavaScript - Stack Overflow
Jun 10, 2010 · The best way in my opinion is to use the Array.forEach function. If you cannot use that I would suggest to get the polyfill from MDN. To make it available, it is certainly the safest way to iterate over an array in JavaScript. Array.prototype.forEach() So as others has suggested, this is almost always what you want:
Loop (for each) over an array in JavaScript - Stack Overflow
Feb 17, 2012 · In JavaScript, there are many ways of accomplishing both of these goals. However, some are more convenient than others. Below you can find some commonly used methods (the most convenient IMO) to accomplish array …
What's the fastest way to loop through an array in JavaScript?
Mar 18, 2011 · This is very different from iterating the elements of an array, has no guaranteed order, will include the length property, and will likely lead to worse performance. It's simply not meant to be used for array iteration. Furthermore, the code you wrote will only run the inner function for the enumerable properties whose values are truthy. –
Javascript: (ES5) Definitive guide to array iteration
Nov 7, 2013 · There is so much confusion about how to "correctly" iterate over an Array in Javascript. There are at least two posts with several hundred upvotes, but the answers are very contradicting. I'm confused. ES5 added the Array.prototype.forEach function. Is this the final answer or are there still pitfalls to avoid stepping in?
javascript - How to loop through an array containing objects and …
The console should bring up every object in the array, right? But in fact it only displays the first object. if I console log the array outside of the loop, all the objects appear so there's definitely more in there. Anyway, here's the next problem. How do I access, for example Object1.x in the array, using the loop?
javascript - Using async/await with a forEach loop - Stack Overflow
Jun 2, 2016 · // Example of asyncForEach Array poly-fill for NodeJs // file: asyncForEach.js // Define asynForEach function async function asyncForEach(iteratorFunction){ let indexer = 0 for(let data of this){ await iteratorFunction(data, indexer) indexer++ } } // Append it as an Array prototype property Array.prototype.asyncForEach = asyncForEach module ...
How to iterate over an array and remove elements in JavaScript
Feb 27, 2017 · The problem is that JavaScript doesn't seem to have a for each loop and if I use a for loop I run into problems with it basically trying to check elements beyond the bounds of the array, or missing elements in the array because the indexes change.
javascript - catch forEach last iteration - Stack Overflow
Apr 20, 2015 · Ask questions, find answers and collaborate at work with Stack Overflow for Teams. Try Teams for free Explore Teams
Get loop counter/index using for…of syntax in JavaScript
for (let [index, val] of array.entries()) { // your code goes here } Note that Array.entries() returns an iterator, which is what allows it to work in the for-of loop; don't confuse this with Object.entries(), which returns an array of key-value pairs.
Go to "next" iteration in JavaScript forEach loop
Jul 14, 2015 · JavaScript's forEach works a bit different from how one might be used to from other languages for each loops. If reading on the MDN, it says that a function is executed for each of the elements in the array, in ascending order. To continue to the next element, that is, run the next function, you can simply return the current function without ...