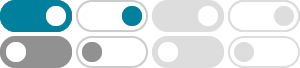
javascript - How to append something to an array ... - Stack Overflow
Dec 9, 2008 · It will add a new element at the end of the array. But if you intend to add multiple elements then store the elements in a new array and concat the second array with the first array...either way you wish. arr=['a','b','c']; arr.push('d'); //now print the array in console.log and it will contain 'a','b','c','d' as elements. console.log(array);
Append an array to another array in JavaScript - Stack Overflow
If you want to modify the original array instead of returning a new array, use .push()... array1.push.apply(array1, array2); array1.push.apply(array1, array3); I used .apply to push the individual members of arrays 2 and 3 at once.
javascript - How to insert an item into an array at a specific index ...
Feb 25, 2009 · I am looking for a JavaScript array insert method, in the style of: arr.insert(index, item) Preferably in jQuery, but any JavaScript implementation will do at this point.
How can I add new array elements at the beginning of an array in ...
Actually, all unshift/push and shift/pop mutate the source array. The unshift/push add an item to the existed array from begin/end and shift/pop remove an item from the beginning/end of an array. But there are few ways to add items to an array without a mutation. the result is a new array, to add to the end of array use below code:
Adding a new array element to a JSON object - Stack Overflow
Sep 19, 2013 · It more clearly delineates the boundaries between operating on the javascript objects and the JSON text representation of those objects. I think it is essential in understanding that once the JSON has been parsed, we are operating on pure javascript objects -- the fact that they originally came from JSON is irrelevant.
javascript - How to add an array of values to a Set - Stack Overflow
The old school way of adding all values of an array into the Set is: // for the sake of this example imagine this set was created somewhere else // and I cannot construct a new one out of an array...
how to add array element values with javascript?
Oct 8, 2013 · Sounds like your array elements are Strings, try to convert them to Number when adding: var total = 0; for (var i=0; i<10; i++){ total += +myArray[i]; } Note that I use the unary plus operator ( +myArray[i] ), this is one common way to make sure you are adding up numbers, not concatenating strings.
javascript - Adding elements to object - Stack Overflow
Jan 9, 2013 · Your element is not an array, however your cart needs to be an array in order to support many element objects. Code example: var element = {}, cart = []; element.id = id; element.quantity = quantity; cart.push(element); If you want cart to be an array of objects in the form { element: { id: 10, quantity: 1} } then perform:
Add new value to an existing array in JavaScript - Stack Overflow
Jan 4, 2010 · Using .push() is the better way to add to an array, since you don't need to know how many items are already there, and you can add many items in one function call. Share Improve this answer
Javascript - insert an array inside another array - Stack Overflow
@icCube: I just ran a benchmark, comparing this method to that in patrick's answer. It starts with a1 and a2 as in the example, and injects the a2 variable into a1 10,000 times (so that in the end, you have an array with 20,005 elements).