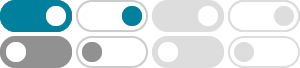
Python | Sort a Dictionary - GeeksforGeeks
Apr 12, 2023 · this approach uses the sorted () function to sort a dictionary by its values in either ascending or descending order. The sorted () function is called with the items () method of the dictionary and a key function that returns the second element of …
Sorting a Python Dictionary: Values, Keys, and More
Dec 14, 2024 · To sort a Python dictionary by its keys, you use the sorted() function combined with .items(). This approach returns a list of tuples sorted by keys, which you can convert back to a dictionary using the dict() constructor.
python - How do I sort a dictionary by key? - Stack Overflow
Jan 26, 2017 · A simple way I found to sort a dictionary is to create a new one, based on the sorted key:value items of the one you're trying to sort. If you want to sort dict = {} , retrieve all its items using the associated method, sort them using …
python - How do I sort a dictionary by value? - Stack Overflow
Mar 5, 2009 · It is not possible to sort a dictionary, only to get a representation of a dictionary that is sorted. Dictionaries are inherently orderless, but other types, such as lists and tuples, are not. So you need an ordered data type to represent sorted values, which will be a list—probably a list of tuples. For instance,
How to sort a list of dictionaries by a value of the dictionary in Python?
Alternatively, you can use operator.itemgetter instead of defining the function yourself. For completeness, add reverse=True to sort in descending order. Using key is not only cleaner but more effecient too. The fastest way would be to add a newlist.reverse () statement.
Sort Python Dictionary by Value - GeeksforGeeks
Jan 23, 2024 · In this article, we'll explore five different methods to sort a Python dictionary by its values, along with simple examples for each approach. Sort Python Dictionary by Value. Below, are the example of Sort Python Dictionary by Value in Python. Using a For Loop; Using sorted() Method; Using the itemgetter() Return New Dictionary with Sorted Value
Sort Python Dictionary by Key or Value - GeeksforGeeks
Oct 14, 2024 · We can sort a dictionary by values using these methods: First, sort the keys alphabetically using key_value.iterkeys () function. Second, sort the keys alphabetically using the sorted (key_value) function & print the value corresponding to it. We have covered different examples based on sorting dictionary by key or value.
How to Sort a Dictionary by Key or Value in Python
Jan 23, 2025 · Learn how to sort Python dictionaries by values or keys. In this tutorial, we'll cover built-in functions like `sorted()` and `dict.items()` to help you sort dictionaries in ascending or descending order and handle edge cases using `OrderedDict`.
Sorting Dictionaries in Python: A Comprehensive Guide
Feb 20, 2025 · In this blog post, we will explore different ways to sort a dictionary in Python, covering the basic concepts, usage methods, common practices, and best practices. In Python, dictionaries are unordered collections, which means that the order of the key-value pairs is …
How to Sort a Dictionary by Value in Python? - Python Guides
Feb 19, 2025 · In this tutorial, I explained how to sort a dictionary by value in Python. We explored different approaches to accomplish this, including using the sorted() function with lambda expressions and the itemgetter() function from the operator module.