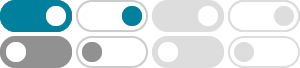
How do I get time of a Python program's execution?
Oct 13, 2009 · I've looked at the timeit module, but it seems it's only for small snippets of code. I want to time the whole program. $ python -mtimeit -n1 -r1 -t -s "from your_module import main" "main()" It runs your_module.main() function one time and print the elapsed time using time.time() function as a timer.
python - How to call a script from another script? - Stack Overflow
Running Python as a subprocess of Python is almost never the correct solution. If you do go with a subprocess, you should avoid Popen unless the higher-level functions really cannot do what you want. In this case, check_call or run would do everything you need and more, with substantially less plumbing in your own code. –
python - How to repeatedly execute a function every x seconds?
This code will run as a daemon and is effectively like calling the python script every minute using a cron, but without requiring that to be set up by the user. In this question about a cron implemented in Python , the solution appears to effectively just sleep() for x seconds.
How do I execute a program or call a system command?
Note on Python version: If you are still using Python 2, subprocess.call works in a similar way. ProTip: shlex.split can help you to parse the command for run, call, and other subprocess functions in case you don't want (or you can't!) provide them in form of lists: import shlex import subprocess subprocess.run(shlex.split('ls -l'))
How can I make one python file run another? - Stack Overflow
Using import adds namespacing to the functions, e.g. function() becomes filename.function(). To avoid this use "from name import *". This will also run the code body. Running with os.system() will not keep the defined function (as it was run in another process). execfile is exec() in Python 3, and it doesn't work. –
Key shortcut for running python file in VS code - Stack Overflow
Apr 27, 2022 · The button just runs the file in a Python terminal, the default command associated with Ctrl+F5 will run the file in the Python debugger, just with the debugging turned off. – Ajean Commented Nov 22, 2023 at 18:04
multithreading - Creating Threads in python - Stack Overflow
May 9, 2019 · Pygame + python: 1 part of code has pygame.wait while rest of code runs 2 Is there a way to make a loop that runs in the background while other code continues to run in python?
How to execute Python code from within Visual Studio Code
May 1, 2015 · First: To run code: use shortcut Ctrl + Alt + N; or press F1 and then select/type Run Code, or right click in a text editor window and then click Run Code in the editor context menu; or click the Run Code button in editor title menu (triangle to the right) or click Run Code in context menu of file explorer. Second: To stop the running code:
How to Execute a Python Script in Notepad++? - Stack Overflow
Links: ) This works fine, and you can even run files in interactive mode by adding -i to your code (links: ). Run python program in IDLE with code something like this (links: , in these links C:\Path\to\Python\Lib\idlelib\idle.py is used, but I am using C:\Path\to\Python\Lib\idlelib\idle.bat instead, because idle.bat sets the right current ...
python - Run function from the command line - Stack Overflow
It is always an option to enter python on the command line with the command python. then import your file so import example_file. then run the command with example_file.hello() This avoids the weird .pyc copy function that crops up every time you run python -c etc.