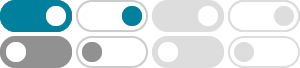
Reverse words in python using list - Stack Overflow
May 28, 2014 · You can do this directly using the indexing syntax in Python. Try: word_inverted = word[-1::-1] This syntax means "start at the last letter in word (index -1), move backwards one …
string - How to reverse words in Python - Stack Overflow
Sep 18, 2013 · Then, may be you are on python 3 - use range() instead of xrange(). Use the slice notation: >>> string = "Hello world." You can read more about the slice notatoin here. A string …
Python: How do I reverse every character in a string list?
There are two things you need to use, the reverse function for lists, and the [::-1] to reverse strings. This can be done as a list comprehension as follows. myList.reverse newList = [x[::-1] …
Reverse All Strings in String List in Python - GeeksforGeeks
Dec 27, 2024 · In this article, we will explore various methods to reverse all strings in string list in Python. The simplest way to do is by using a loop. We can use a for loop to iterate over the list …
Reverse Words in a Given String in Python - GeeksforGeeks
Jan 6, 2025 · Using split () and join () is the most common method to reverse the words in a string. The split() method splits the string into a list of words. [::-1] reverses the list of words. …
Reversing a List in Python - GeeksforGeeks
Oct 15, 2024 · In this article, we are going to explore multiple ways to reverse a list. Python provides several methods to reverse a list using built-in functions and manual approaches. …
Reverse Words in a Given String in Python - Online Tutorials …
Oct 23, 2019 · 1. Initialize the string. 2. Split the string on space and store the resultant list in a variable called words. 3. Reverse the list words using reversed function. 4. Convert the result …
How to Reverse a String in Python - W3Schools
There is no built-in function to reverse a String in Python. The fastest (and easiest?) way is to use a slice that steps backwards, -1. Reverse the string "Hello World": We have a string, "Hello …
Reverse a list, string, tuple in Python (reverse, reversed)
Aug 16, 2023 · In Python, you can reverse a list using the reverse() method, the built-in reversed() function, or slicing. To reverse a string (str) and a tuple, use reversed() or slicing. Reverse a …
Python String Reversal
String reversal is a common programming task that can be approached in multiple ways in Python. This comprehensive guide will explore various methods to reverse a string, discussing …