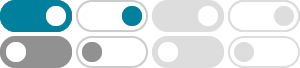
Reading json files in C++ - Stack Overflow
Aug 25, 2015 · Here is another easier possibility to read in a json file: #include "json/json.h" std::ifstream file_input("input.json"); Json::Reader reader; Json::Value root; reader.parse(file_input, root); cout << root; You can then get …
How do I read/write JSON with c++? - Stack Overflow
Jan 12, 2022 · Using a library, it can be done quite easily: // read file. auto json = nlohmann::json::parse("{\"value1\": \"string\"}"); // mutate the json. json["value1"] = "new string"; // write to a stream, or the same file. std::cout << json; // print the json. C++ don't have the built-ins for dealing with json.
RapidJSON – File Read/Write in C++ - GeeksforGeeks
Feb 23, 2023 · RapidJSON is a C++ library for parsing and generating JSON (JavaScript Object Notation) data. It is designed for high performance and can handle very large JSON documents. RapidJSON supports both reading and writing JSON data, as well as validation and manipulation of JSON objects.
How to Read JSON File in C++ - Delft Stack
Feb 2, 2024 · The following code snippet will explain how to read JSON files in C++. ifstream file("file.json"); . Json::Value actualJson; . Json::Reader reader; // Using the reader, we are parsing the json file . reader.parse(file, actualJson); // The actual json has the json data .
How to Read or Fetch JSON File in C++ - CodeSpeedy
In this tutorial, we will learn how to read a JSON file, read data from a JSON file in C++ using boost library and its sublibrary property tree.
Using JSON in C++ | A Practical Guide | StudyPlan.dev
In this lesson, we’ll introduce the JSON format, and how to use it in C++. We’ll be using a popular third-party library, nlohmann::json, which lets us work much more quickly than trying to do everything ourselves.
Working with JSON Data in C++: Complete Guide and Examples
How to set up and use a JSON library in C++. Parsing and generating JSON data. Handling different JSON data types and nested structures. Best practices and common pitfalls. Error handling and debugging techniques. Basic knowledge of C++ (C++11 or later). Familiarity with JSON syntax and data structures. A C++ compiler (e.g., GCC or Clang).
[C++] Making a Simple JSON Parser from Scratch - DEV Community
Jul 30, 2023 · First, our parser requires an I/O system: we read text from the JSON file as an input, and as an output we return JsonValue data to the caller function. To achieve this, we define ReadFile() that reads a text file and returns a std::string object.
nlohmann/json: JSON for Modern C++ - GitHub
In languages such as Python, JSON feels like a first class data type. We used all the operator magic of modern C++ to achieve the same feeling in your code. Check out the examples below and you'll know what I mean. Trivial integration. Our whole code consists of a single header file json.hpp. That's it.
Parsing complex Json file in C++ using JsonCpp - Medium
Jun 13, 2020 · JsonCpp is a lightweight library which is not dependent on any third party. It has a very simple to use interface with single header files using CPP containers. Once parsed by this library the...