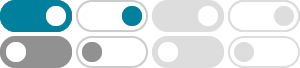
Printing Pyramid Patterns in Python - GeeksforGeeks
Mar 3, 2025 · # Function to print inverted half pyramid pattern def inverted_half_pyramid (n): for i in range (n, 0,-1): for j in range (1, i + 1): print ("* ", end = "") print (" \r ") # Example: Inverted Half Pyramid with n = 5 n = 5 inverted_half_pyramid (n)
Python Program to Create Pyramid Patterns
In this example, you will learn to print half pyramids, inverted pyramids, full pyramids, inverted full pyramids, Pascal's triangle, and Floyd's triangle in Python Programming.
Reverse Pyramid Pattern in Python
Mar 30, 2023 · Python Program to print a Reverse Pyramid Pattern or Reverse Triangle Pattern. Run 3 for loops, one main for row looping, and the other 2 sub-loops for column looping, in the first loop, loop through the range of the triangle for row looping. That is it for this tutorial.
python - Print an n level reverse pyramid as follows where n is …
Aug 17, 2020 · Print an n level reverse pyramid as follows where n is a positive integer taken as input. Each triangle will be made out of “*” symbols and the spaces will be filled by "#" symbols for each row. For example, a 3 level reverse pyramid will look as follows: ***** #***# ##*## Note: There should be no space between the stars.
Python Programs to Print Patterns – Print Number, Pyramid, Star ...
Sep 3, 2024 · Let’s see how to print reversed pyramid pattern in Python. Pattern: – * * * * * * * * * * * * * * * * * * * * * Program:
Printing Inverted Pyramid in Python - Stack Overflow
Nov 25, 2020 · def inverted_pyramid(height): for h in reversed(range(height)): print(" " * (height - h - 1), end = "") n = (1 + h ** 2) % 10 for w in range(1 + h * 2): print(n, end = "") n += 1 if n == 10: n = 0 print() inverted_pyramid(5)
Printing a pyramid pattern inverse order in python
Print an n level reverse pyramid as follows where n is a positive integer taken as input
Python Program to Print Reverse Pyramid Pattern - Java Guides
This Python program prints a reverse pyramid pattern using stars (*). The program uses nested loops to control the number of spaces and stars, ensuring the stars are printed in decreasing order to form the reverse pyramid shape.
Python Program to Print Reverse Pyramid of Numbers
Apr 26, 2024 · Given the number of rows of the pyramid, the task is to print a Reverse Pyramid of Numbers in C, C++, and Python. Examples: Example1: Input: Given number of rows = 10. Output: 1 2 1 3 2 1 4 3 2 1 5 4 3 2 1 6 5 4 3 2 1 7 6 5 4 3 2 1 8 7 6 5 4 3 2 1 9 8 7 6 5 4 3 2 1. Python Program to Print Square Pattern with Numbers
Print Reverse Pyramid || Patterns || Python - Help For Coders
In this we are going to see a basic program to Print Reverse Pyramid in Python Programming Language. Copy code n = int ( input ( "Enter a number: " )) for i in range ( 1 , n): for j in range ( 1 , n-i): print( " " , end= " " ) for k in range (i, 0 , - 1 ): print(k, end= " " ) for l in range ( 2 , i+ 1 ): print(l, end= " " ) print() # Coded By ...