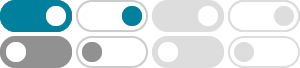
How do I set a function parameter as a string in python?
Jun 23, 2016 · If you want a parameter as a string or float or etc, try this: z = x + y. return z. In the case of a string, it is "y: str". This is a complete version to use input () string = input() # <- user can type string into command line and end by 'Enter' count = 0. for each in string: if each == letter: count += 1. print (count) 4 # <- result.
Initialize a string variable in Python: "" or None?
Another way to initialize an empty string is by using the built-in str() function with no arguments. str(object='') Return a string containing a nicely printable representation of an object.
python - define function with strings as parameter - Stack Overflow
Because word isn't explicitly defined as a string in the function, it's just a variable. Passing 'abc' into the function means word gets assigned a value of 'abc'. It's because function parameters are also variables. If you enclose the string parameter for a function in quotation marks, e.g. "word", then it's not a parameter anymore.
Python Strings - W3Schools
Strings in python are surrounded by either single quotation marks, or double quotation marks. 'hello' is the same as "hello". You can display a string literal with the print() function: You can use quotes inside a string, as long as they don't match the quotes surrounding the string:
Python Function Examples – How to Declare and Invoke with Parameters
Aug 24, 2021 · To execute the code inside the function, you have make a function invokation or else a function call. You can then call the function as many times as you want. To call a function you need to do this:
Python Strings: An In-Depth Tutorial (55+ Code Examples)
Apr 21, 2022 · We can declare a Python string using a single quote, a double quote, a triple quote, or the str() function. The following code snippet shows how to declare a string in Python: # A single quote string single_quote = 'a' # This is an …
How to Declare & Initialise a String in different ... - GeeksforGeeks
Nov 11, 2023 · To declare and initialize a string in different programming languages, you can use language-specific syntax and functions. Below are examples in C++, C#, Python, JavaScript, and Java, with explanations for each language: Steps: Include the necessary header files ( #include<string> ). Declare a string variable using the std::string type.
What are the Different ways to Create Strings in Python - Python …
Apr 9, 2024 · How to Declare String in Python. You can declare the string in Python using the single, double, and triple single or double quotes that you have used in the above sections. Declaring means defining it with a variable name and assigning a string, as shown in the code below. str_name = " " | ' ' | ''' ''' | """ """" Where,
Python String - GeeksforGeeks
Mar 10, 2025 · Python provides a various built-in methods to manipulate strings. Below are some of the most useful methods. len (): The len () function returns the total number of characters in a string. upper () and lower (): upper () method converts all characters to uppercase. lower () method converts all characters to lowercase.
Python Variables: How to Define/Declare String Variable Types
Jan 24, 2024 · These examples cover the basics of defining and manipulating string variables in Python. Remember that Python is dynamically typed, so you don’t need to explicitly declare the variable type, and you can easily perform various operations on strings using built-in methods.