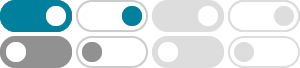
JavaScript Arrays - W3Schools
Using an array literal is the easiest way to create a JavaScript Array. Syntax: const array_name = [item1, item2, ...]; It is a common practice to declare arrays with the const keyword. Learn …
JavaScript - Convert a Number into JS Array - GeeksforGeeks
Jan 10, 2025 · In JavaScript, there are various ways to transform a number into an array of its digits. This can be useful in scenarios like performing calculations on each digit, validating …
javascript - How to create an array containing 1...N - Stack Overflow
This is probably the fastest way to generate an array of numbers. Shortest. var a=[],b=N;while(b--)a[b]=b+1; Inline. var arr=(function(a,b){while(a--)b[a]=a;return b})(10,[]); …
javascript - How to convert an integer into an array of digits
Oct 4, 2013 · The code line Array.from(String(numToSeparate), Number); will convert the number into a string, take each character of that string, convert it into a number and put in a new array. …
JavaScript – Create an Array Containing 1…N Numbers
Dec 31, 2024 · Here are some methods to create an array containing 1…N numbers. 1. Using for Loop. The for loop iterates from 1 to N, adding each number to the array. 1, 2, 3, 4, 5, 6, 7, 8, …
JavaScript Arrays - How to Create an Array in JavaScript
May 16, 2022 · In this article I showed you three ways to create an array using the assignment operator, Array constructor, and Array.of() method. The most common way to create an array …
javascript - ES6 - generate an array of numbers - Stack Overflow
Oct 7, 2016 · function _toConsumableArray(arr) { if (Array.isArray(arr)) { for (var i = 0, arr2 = Array(arr.length); i < arr.length; i++) { arr2[i] = arr[i]; } return arr2; } else { return Array.from(arr); …
Array() constructor - JavaScript | MDN - MDN Web Docs
Oct 4, 2023 · Arrays can be created using a constructor with a single number parameter. An array is created with its length property set to that number, and the array elements are empty slots.
JavaScript Arrays - GeeksforGeeks
Feb 10, 2025 · Arrays in JavaScript are zero-indexed, meaning the first element is at index 0, the second at index 1, and so on. 1. Create Array using Literal. Creating an array using array …
JavaScript Array Handbook – Learn How JS Array Methods Work …
Aug 31, 2023 · How to Create an Array in JavaScript. There are two ways you can create an array in JavaScript: Using the square brackets [] Using the Array() constructor; The square brackets …