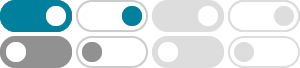
python - How to convert ListNode from LeetCode to regular list…
Sep 24, 2021 · So i decided to convert it into list. Please help me how to do it, how to iterate through all ListNode and put all of it values to the regular list and then vice versa: list -> ListNode in order to return my answer in correct type.
python - Converting a List into ListNode - Stack Overflow
Aug 8, 2019 · Below is LeetCode's implementation of a LinkedList and my way of converting a list into a LinkedList # Definition for singly-linked list. # class ListNode(object): # def __init__(self, x): # self.val = x # self.next = None list1 = [4,5,1,2,0,4] head = ListNode(list1[0]) e = 1 while e < len(list1): print(head) head.next = ListNode(list1[e]) head ...
python - Converting a list to a linked list - Stack Overflow
Jul 22, 2015 · I already have a class for the link but I'm trying to figure out how to convert a list to linked list, for example: def list_to_link(lst): """Takes a Python list and returns a Link with the same elements.
Python | Convert list into list of lists - GeeksforGeeks
May 8, 2023 · Convert the numpy array into a list of lists using the tolist() method. Return the resulting list of lists from the function. Define a list lst with some values. Call the convert_to_list_of_lists function with the input list lst and store the result in a variable named res. Print the result res.
Convert List to Linked List & Vice-Versa in Python (2 Examples)
In this first example, we will convert the list into a linked list. Therefore, run the code below: def __init__(self, val = 0, next = None): self. val = val. self. next = next. # create first node as head . # loop through list to create nodes and link them together for item in my_list [1:]: current. next = NodeList (item) .
Create linked list from a given array - GeeksforGeeks
Aug 1, 2024 · Given an array arr [] of size N. The task is to create linked list from the given array. Simple Approach: For each element of an array arr [] we create a node in a linked list and insert it at the end. Efficient Approach: We traverse array from end and insert every element at the beginning of the list. Time Complexity : O (n)
Convert a Singly Linked List to an array - GeeksforGeeks
Sep 4, 2024 · Given a singly linked list and the task is to convert it into an array. Examples: Input: List = 1 -> 2 -> 3 -> 4 -> NULL Output: 1 2 3 4. Input: List = 10 -> 20 -> 30 -> 40 -> 50 -> NULL Output: 10 20 30 40 50 . Approach: The idea is to iterate through the linked list and for each node add its value into the array. C++
What is a List Node in Python? (2 Examples)
This tutorial demonstrates what a list node is in the Python programming language - Create sample linked list structure - Python coding
Python: Converting a linked list to a list using a list …
Feb 20, 2019 · Let's say I have a simple linked list implementation using a dictionary called child that associates a node with the following node in the linked list. For example: a->b->c->d Would be : {a:b,b:c,c:d,d:None} Converting this to a normal list is trivial, myList=[] node=a while node!=None: myList.append(node) node=child[node]
Are ListNodes/LinkedLists even a thing in Python? How the hell ... - Reddit
Dec 6, 2023 · def addTwoNumbers(self, l1, l2): """ :type l1: ListNode. :type l2: ListNode. :rtype: ListNode. """ list1=[] list2=[] def addtolist(node, alist): while node.next: alist.append(str(node.val)) return addtolist(node.next, alist) else: return alist.append(str(node.val)) addtolist(l1, list1) addtolist(l2, list2) int1 = int("".join(list1))