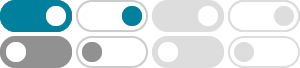
How can I determine a Python variable's type? - Stack Overflow
Dec 31, 2008 · How to determine the variable type in Python? So if you have a variable, for example: one = 1 You want to know its type? There are right ways and wrong ways to do just about everything in Python. Here's the right way: Use type >>> type(one) <type 'int'> You can use the __name__ attribute to get the name of the object.
What's the canonical way to check for type in Python?
In Python, you can use the built-in isinstance() function to check if an object is of a given type, or if it inherits from a given type. To check if the object o is of type str, you would use the following code: if isinstance(o, str): # o is of type str You can also use type() function to check the object type. if type(o) == str: # o is of type str
python - Determine the type of an object? - Stack Overflow
Feb 9, 2010 · Determine the type of a Python object. Determine the type of an object with type >>> obj = object() >>> type(obj) <class 'object'> Although it works, avoid double underscore attributes like __class__ - they're not semantically public, and, while perhaps not in this case, the builtin functions usually have better behavior.
python - Identifying the data type of an input - Stack Overflow
Mar 5, 2014 · I'm using Python 3.2.3 and I know I could use type() to get the type of the data but in Python all user inputs are taken as strings and I don't know how to determine whether the input is a string or Boolean or integer or float. Here is that part of the code:
How to compare type of an object in Python? - Stack Overflow
# check if x is a regular string type(x) == str # check if x is either a regular string or a unicode string type(x) in [str, unicode] # alternatively: isinstance(x, basestring) # check if x is an integer type(x) == int # check if x is a NoneType x is None Note the last case: there is only one instance of NoneType in python, and that is None.
python - How to check if type of a variable is string ... - Stack …
Jan 30, 2011 · # Option 1: check to see if `my_variable` is of type `str` type(my_variable) is str # Option 2: check to see if `my_variable` is of type `str`, including # being a subclass of type `str` (ie: also see if `my_variable` is any object # which inherits from `str` as a parent class) isinstance(my_variable, str)
python - Checking whether a variable is an integer or not - Stack …
Aug 17, 2010 · This adheres to Python's strong polymorphism: you should allow any object that behaves like an int, instead of mandating that it be one. BUT. The classical Python mentality, though, is that it's easier to ask forgiveness than permission. In other words, don't check whether x is an integer; assume that it is and catch the exception results if it ...
Python how to check the type of a variable - Stack Overflow
Feb 27, 2018 · Basically, I need to check the type of data of which a variable is storing before replacing the data stored in the variable with the new data. For example, how to check if the variable is storing a string data or an integer data? Source Code:
python - pandas how to check dtype for all columns in a …
Nov 1, 2016 · The singular form dtype is used to check the data type for a single column. And the plural form dtypes is for data frame which returns data types for all columns. Essentially: For a single column: dataframe.column.dtype For all columns: dataframe.dtypes Example:
python - check type within numpy array - Stack Overflow
The bytes in the data buffer do not have a type, because they are not Python objects. The array has a dtype parameter, which is used to interpret those bytes. If dtype is int32 (there are various synonyms), 4 bytes are interpreted as an integer.