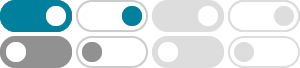
python - How to modify list entries during for loop? - Stack Overflow
Sep 8, 2023 · No you wouldn't alter the "content" of the list, if you could mutate strings that way. But in Python they are not mutable. Any string operation returns a new string. If you had a list …
python - How do I convert all of the items in a list to floats? - Stack ...
float(item) do the right thing: it converts its argument to float and and return it, but it doesn't change argument in-place. A simple fix for your code is: new_list = [] for item in list: …
python - How can I reorder a list? - Stack Overflow
If set to True, then the list elements are sorted as if each comparison were reversed. In general, the key and reverse conversion processes are much faster than specifying an equivalent cmp …
python - How to convert list to string - Stack Overflow
Apr 11, 2011 · Agree with @Bogdan. This answer creates a string in which the list elements are joined together with no whitespace or comma in between. You can use ', '.join(list1) to join the …
python: replace elements in list with conditional
Sep 21, 2015 · In python(2.x), comparison of a list with an int will compare the types, not the values. So, your comparison results in True which is equivalent to 1 . In other words, your …
Python - How to change values in a list of lists? - Stack Overflow
Dec 6, 2012 · item itself is a copy too, but it is a copy of a reference to the original nested list, so when you refer to its elements the original list is updated. This isn't as convenient since you …
Python change type of whole list? - Stack Overflow
a=input()#taking input as string. Like this-10 5 7 1(in one line) a=a.split()#now splitting at the white space and it becomes ['10','5','7','1'] #split returns a list for i in range(len(a)): a[i]=int(a[i]) #now …
How to switch position of two items in a Python list?
Python: change elements position in a list of instances. 3. Reorder Python List. 4. Swap two values ...
python - Convert all strings in a list to integers - Stack Overflow
There are several methods to convert string numbers in a list to integers. In Python 2.x you can use the map function: >>> results = ['1', '2', '3'] >>> results = map(int, results) >>> results [1, 2, …
python - Move an item inside a list? - Stack Overflow
Oct 31, 2013 · Use the insert method of a list: l = list(...) l.insert(index, item) Alternatively, you can use a slice notation: l[index:index] = [item] If you want to move an item that's already in the list …