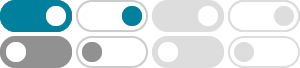
How to Get a List as User Input in Python
Dec 15, 2021 · In this article, we will discuss two ways to get a list as user input in Python. We can get a list of values using the for loop in python. For this, we can first create an empty list and a count variable. After that, we will ask the user for the total count of values in the list.
python - How to let the user select an input from a finite list ...
One of inquirer's feature is to let users select from a list with the keyboard arrows keys, not requiring them to write their answers. This way you can achieve better UX for your console application. Here is an example taken from the documentation:
python - Get a list of numbers as input from the user - Stack Overflow
Get a list of number as input from the user. This can be done by using list in python. L=list(map(int,input(),split())) Here L indicates list, map is used to map input with the position, int specifies the datatype of the user input which is in integer datatype, and split() is used to split the number based on space..
python - How do I store user input into a list? - Stack Overflow
Apr 4, 2015 · you can do it in a single line by using functional programming construct of python called map, python2. input_list = map(int, raw_input().split()) python3. input_list = map(int, input().split())
Python take a list as input from a user - PYnative
Sep 8, 2023 · Below are the simple steps to input a list of numbers in Python. Use an input() function. Use an input() function to accept the list elements from a user in the format of a string separated by space. Use the split() function of the string class. Next, the split() method breaks an input string into a list. In Python, the split() method divides
5 Best Ways to Get a List as Input from User in Python
Mar 11, 2024 · import ast list_str = input("Enter the list using Python's list syntax: ") number_list = ast.literal_eval(list_str) print(number_list) If a user enters the valid Python list [25, 50, 100] , the output will be:
How to Ask for User Input in Python: A Complete Guide
To ask for user input in Python, use the built-in input() function. For example: Output example: In addition to asking for simple string input like this, you also want to learn how to: Ask for multiple inputs in one go. Ask for input again until valid input is …
Get a List as Input from User in Python - Online Tutorials Library
Jul 9, 2020 · Learn how to get a list as input from the user in Python with step-by-step examples and explanations.
Python Take list as an input from a user - Techgeekbuzz
Feb 11, 2025 · This article is a step-by-step guide on how to take the list as input from a user in Python using the input() method, along with examples.
In Python, how to ask for user to input a list, and then to be …
Dec 1, 2015 · A first solution would be to ask the user for the number of elements in the list, and then ask for each element individually in a for loop. Another solution, if the user has to input a list of int, would be to require him to write them separated by commas.