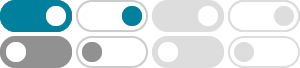
python - Adding dictionaries together - Stack Overflow
Here are quite a few ways to add dictionaries. You can use Python3's dictionary unpacking feature: ndic = {**dic0, **dic1} Note that in the case of duplicates, values from later arguments are used. This is also the case for the other examples listed here.
How do I merge two dictionaries in a single expression in Python?
How can I merge two Python dictionaries in a single expression? For dictionaries x and y, their shallowly-merged dictionary z takes values from y, replacing those from x. In Python 3.9.0 or greater (released 17 October 2020, PEP-584, discussed here): z = x | y In Python 3.5 or greater: z = {**x, **y} In Python 2, (or 3.4 or lower) write a function:
python - Add values from two dictionaries - Stack Overflow
Aug 25, 2018 · How can I add values in different dictionaries with same keys together? 549 Is there any pythonic way to combine two dicts (adding values for keys that appear in both)?
python - Append a dictionary to a dictionary - Stack Overflow
update() accepts either another dictionary object or an iterable of key/value pairs (as tuples or other iterables of length two). If keyword arguments are specified, the dictionary is then updated with those key/value pairs: d.update(red=1, blue=2).
dictionary - How do I merge dictionaries together in Python?
May 9, 2010 · Starting in Python 3.9, the operator | creates a new dictionary with the merged keys and values from two dictionaries: # d1 = { 'a': 1, 'b': 2 } # d2 = { 'b': 1, 'c': 3 } d3 = d2 | d1 # d3: {'b': 2, 'c': 3, 'a': 1} This: Creates a new dictionary d3 with the merged keys and values of d2 and d1. The values of d1 take priority when d2 and d1 share ...
python - How to concatenate two dictionaries to create a new one ...
Unless all keys are known to be strings, option 2 is an abuse of a Python 2 implementation detail (the fact that some builtins implemented in C bypassed the expected checks on keyword arguments). In Python 3 (and in PyPy), option 2 will fail with non-string keys. –
How do I combine two lists into a dictionary in Python?
Apr 4, 2013 · dict can take an iterable of iterables, where each inner iterable has two items -- it then uses the first as the key and the second as the value for each item. Share Improve this answer
python - How can I add new keys to a dictionary? - Stack Overflow
Jun 21, 2009 · @hegash the d[key]=val syntax as it is shorter and can handle any object as key (as long it is hashable), and only sets one value, whereas the .update(key1=val1, key2=val2) is nicer if you want to set multiple values at the same time, as long as the keys are strings (since kwargs are converted to strings).
dictionary - How to merge and sum of two dictionaries in Python ...
I have a dictionary below, and I want to add to another dictionary with not necessarily distinct elements and merge its results. Is there any built-in function for this, or do I need to make my own...
python - append multiple values for one key in a dictionary - Stack ...
I am new to python and I have a list of years and values for each year. What I want to do is check if the year already exists in a dictionary and if it does, append the value to that list of values for the specific key. So for instance, I have a list of years and have one value for each year: 2010 2 2009 4 1989 8 2009 7