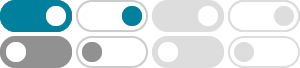
How to find greatest common divisor using recursive function in Python …
Dec 2, 2019 · I am asked to find the greatest common divisor of integers x and y using a recursive function in Python. The condition says that: if y is equal to 0 then gcd (x,y) is x ; otherwise gcd(x,y) is gcd(y,x%y).
Python Program to Find the GCD of Two Numbers using Recursion
Here is source code of the Python Program to find the GCD of two numbers using recursion. The program output is also shown below. if(b ==0): return a. else: return gcd (b, a%b) . 1. User must enter two numbers. 2. The two numbers are passed as arguments to a recursive function. 3. When the second number becomes 0, the first number is returned. 4.
Python Program to Find the Gcd of Two Numbers - GeeksforGeeks
Feb 22, 2025 · The task of finding the GCD (Greatest Common Divisor) of two numbers in Python involves determining the largest number that divides both input values without leaving a remainder. For example, if a = 60 and b = 48, the GCD is 12, as 12 is the largest number that divides both 60 and 48 evenly.
gcd() in Python - GeeksforGeeks
Oct 31, 2022 · The Highest Common Factor (HCF), also called gcd, can be computed in python using a single function offered by math module and hence can make tasks easier in many situations. Naive Methods to compute gcd. Output: If only …
Compute GCD of Two Numbers Recursively in Python - Online …
Oct 12, 2021 · Learn how to compute the GCD of two numbers recursively in Python with this step-by-step guide and example code.
Program to Find GCD or HCF of Two Numbers - GeeksforGeeks
Feb 14, 2025 · # Python program to find GCD of two numbers # Recursive function to return gcd of a and b def gcd (a, b): # Everything divides 0 if (a == 0): return b if (b == 0): return a # Base case if (a == b): return a # a is greater if (a > b): return gcd (a-b, b) return gcd (a, b-a) # Driver code if __name__ == '__main__': a = 98 b = 56 if (gcd (a, b ...
Code for Greatest Common Divisor in Python - Stack Overflow
Jun 24, 2012 · The greatest common divisor (GCD) of a and b is the largest number that divides both of them with no remainder. One way to find the GCD of two numbers is Euclid’s algorithm, which is based on the observation that if r is the remainder when a …
5 Best Ways to Compute GCD of Two Numbers Recursively in Python
Mar 3, 2024 · The GCD is the largest positive integer that divides both numbers without leaving a remainder. For instance, the GCD of 48 and 18 is 6. This article demonstrates how to compute the GCD of two numbers using various recursive methods in Python. Method 1: Euclidean Algorithm Using Subtraction
Calculate the extended gcd using a recursive function in Python
Sep 22, 2012 · Now I am asked to write a recursive function gcd2(a,b) that returns a list of three numbers (g, s, t) where g = gcd(a, b) and g = s*a + t*b. This means that you would enter two values (a and b) into the gcd(a, b) function.
Python Program to find GCD of Two Numbers - Tutorial Gateway
Python Program to find the GCD of Two Numbers using Recursion. It allows the user to enter two positive integer values and calculate the Greatest Common Divisor of those two values by calling the findGreatestCD function recursively.
- Some results have been removed