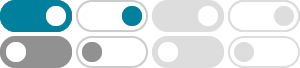
Javascript for loop console print in one line - Stack Overflow
Aug 9, 2010 · We can use the process.stdout.write() method to print to the console without trailing newline. It only takes strings as arguments, but in this case i + " "is a string so it works: for (var i = 1; i < 11; i += 1) { process.stdout.write(i + " "); } Note: It will work only with Node.js.
JavaScript Console Output to Single Line with For and While Loop
Oct 10, 2015 · I need to figure out how to output a list of numbers in a loop to the console. However, the output to the console must be in the same line, as opposed cascading down the page with every new number. Below, I have two separate …
JavaScript for loop (with Examples) - Programiz
In this example, we used the for loop to print "Hello, world!" three times to the console. The syntax of the for loop is: // for loop body . Here, initialExpression - Initializes a counter variable. condition - The condition to be evaluated. If true, the body of the for loop is executed. updateExpression - Updates the value of initialExpression.
loops - How to output to console in real time in Javascript?
Dec 28, 2016 · If you want a smoother output, I would suggest avoiding the for loop, and instead use requestAnimationFrame which will manage when to print out the results. var counter = 0; var max = 100000; function myPrint(){ if(counter < max){ console.log(counter++); requestAnimationFrame(myPrint); } } myPrint();
JavaScript For Loop - W3Schools
JavaScript supports different kinds of loops: The for statement creates a loop with 3 optional expressions: Expression 1 is executed (one time) before the execution of the code block. Expression 2 defines the condition for executing the code block. Expression 3 is executed (every time) after the code block has been executed.
JavaScript Console.log() Example – How to Print to the Console …
Sep 9, 2020 · We can print messages to the console conditionally with console.assert(). If the first argument is false, then the message will be logged. If we were to change isItWorking to true, then the message will not be logged.
JavaScript For Loop – Explained with Examples
May 27, 2022 · For Loop Examples in JavaScript. At this point, we now understand what loops are, so let’s take a look at some examples and see how we can use loops. How to Display Text Multiple Times. Let’s start by displaying some text several times until our condition is met.
JavaScript for Loop By Examples - JavaScript Tutorial
Let’s take some examples of using the for loop statement. The following example uses the for loop statement to show numbers from 1 to 4 to the console: console.log(i); Output: How it works. First, declare a variable counter and initialize it to 1. Second, display the value of counter in the console if counter is less than 5.
JavaScript For Loop - GeeksforGeeks
Nov 19, 2024 · JavaScript for loop is a control flow statement that allows code to be executed repeatedly based on a condition. It consists of three parts: initialization, condition, and increment/decrement. For loop to print table of a number. For loop to print elements of an array.
JavaScript For Loop – Explained with Examples – TheLinuxCode
What Are JavaScript For Loops? A for loop allows you to repeatedly execute a block of code over a fixed number of iterations. This is perfect when you need to perform the same task multiple times. Here are some examples of when for loops come in handy: