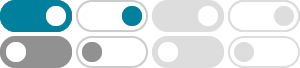
Print the Fibonacci sequence – Python | GeeksforGeeks
Mar 22, 2025 · To print the Fibonacci sequence in Python, we need to generate a series of numbers where each number is the sum of the two preceding ones, starting from 0 and 1. The Fibonacci sequence follows a specific pattern that begins with 0 and 1, and every subsequent number is the sum of the two previous numbers.
5 Best Ways to Compute the Fibonacci Series without Recursion in Python
Mar 7, 2024 · A generator function in Python uses the keyword yield instead of return to provide a result without stopping its execution. This is particularly useful for generating sequences as it allows for iteration over each element without storing the entire sequence in memory.
Fibonacci Series Program in Python - Python Guides
Aug 27, 2024 · In this Python tutorial, we covered several methods to generate the Fibonacci series in Python, including using for loops, while loops, functions, recursion, and dynamic programming. We also demonstrated how to generate the Fibonacci series up to a specific range without using recursion.
Fibonacci Series without Recursion in Python - Sanfoundry
Here is source code of the Python Program to find the fibonacci series without using recursion. The program output is also shown below.
Find Fibonacci Series Without Using Recursion in Python
Mar 12, 2021 · Learn how to find the Fibonacci series without using recursion in Python with this simple guide and example code.
python - An iterative algorithm for Fibonacci numbers - Stack Overflow
Feb 24, 2013 · Non recursive Fibonacci sequence in python. def fibs(n): f = [] a = 0 b = 1 if n == 0 or n == 1: print n else: f.append(a) f.append(b) while len(f) != n: temp = a + b f.append(temp) a = b b = temp print f fibs(10)
python - Efficient calculation of Fibonacci series - Stack Overflow
Aug 11, 2013 · def Fibonacci(n): if n == 0: return 0 elif n == 1: return 1 else: return Fibonacci(n-1) + Fibonacci(n-2) list1 = [x for x in range(39)] list2 = [i for i in list1 if Fibonacci(i) % 2 == 0] The problem's solution can be easily found by printing sum(list2).
Fibonacci Sequence: Iterative Solution in Python
In Python, we can solve the Fibonacci sequence in both recursive as well as iterative ways, but the iterative way is the best and easiest way to do it. The source code of the Python Program to find the Fibonacci series without using recursion is given below.
Designing Code for Fibonacci Sequence without Recursion
So, without recursion, let’s do it. Approach: Create two variables to hold the values of the previous and second previous numbers. Set both variables to 0 to begin. Below is the implementation: 1)C++ implementation of above approach. 2)Python implementation of above approach. Output: Related Programs:
Fibonacci numbers, with an one-liner in Python 3?
+1 for the generator, I've always found it the most elegant and efficient way to represent the Fibonacci sequence in Python. I didn't know that lambda can call itself, but it would be good we can refer lambda with a keyword, without assigning …
- Some results have been removed