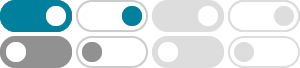
Function for factorial in Python - Stack Overflow
Jan 6, 2022 · The easiest way is to use math.factorial (available in Python 2.6 and above): If you want/have to write it yourself, you can use an iterative approach: fact = 1. for num in range(2, n + 1): fact *= num. return fact. or a recursive approach: if n < 2: return 1. else: return n * factorial(n-1)
Factorial of a Number – Python | GeeksforGeeks
Apr 8, 2025 · In Python, we can calculate the factorial of a number using various methods, such as loops, recursion, built-in functions, and other approaches. Example: Simple Python program to find the factorial of a number
factorial() in Python - GeeksforGeeks
Jul 9, 2024 · Naive method to compute factorial. Using math.factorial () This method is defined in “math” module of python. Because it has C type internal implementation, it is fast. x : The number whose factorial has to be computed. Returns the factorial of desired number. Raises Value error if number is negative or non-integral.
function - Factorial Program in Python using generator - Stack Overflow
Feb 23, 2018 · # factorial function, recursively compute factorial def fact(n): if n==1: # base case return 1 else: # recursive case return n*fact(n-1) # upto desired number computer the factorial function def upto(n): for i in range(1,n+1): yield fact(i) # call function f=upto(3) # print (it will print object not its contents) print(f) # to print use ...
Python lambda function to calculate factorial of a number
Mar 14, 2013 · You infer that the a is... the factorial function. b is the actual parameter. This bit is the application of the factorial: a is the factorial function itself. It takes itself as its first argument, and the evaluation point as the second. This can be generalized to recursive_lambda as long as you don't mind a(a, b - 1) instead of a(b - 1):
Python Program For Factorial (3 Methods With Code) - Python …
To write a factorial program in Python, you can define a function that uses recursion or iteration to calculate the factorial of a number. Here is an example using recursion: def factorial(n): if n == 0: return 1 else: return n * factorial(n-1)
Factorial of a Number in Python - Python Guides
Mar 20, 2025 · This Python tutorial explains, how to print factorial of a number in Python, Python program to print factorial of a number using function, Python program to find factorial of a number using while loop, etc.
Python Factorial Examples - AskPython
Feb 28, 2020 · def factorial(n): if n == 0: # Base case n = 0 return 1 else: # Use the definition of factorial function return n * factorial(n-1) if __name__ == '__main__': print(factorial(4)) print(factorial(7))
Factorial Function in Python: A Comprehensive Guide
Jan 26, 2025 · In Python, implementing the factorial function can be done in multiple ways, each with its own advantages and use cases. This blog post will explore the basic concepts of the factorial function, different methods to implement it in …
Factorial Function In Python: Complete Explanation
Mar 13, 2025 · Factorial Function in Python can be used to calculate a reusable method of factorial of a number. This function is used to return the value of factorial with loops or recursion function which eliminates the need to write programs again and again.