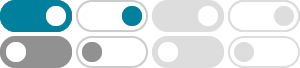
How to initialize a two-dimensional array (list of lists, if not using ...
@codemuncher The reason that [[0] * col] * row doesn't do what you want is because when you initialize a 2d list that way, Python will not create distinct copies of each row. Instead it will init the outer list with pointers to the same copy of [0]*col .
Two dimensional array in python - Stack Overflow
You can first append elements to the initialized array and then for convenience, you can convert it into a numpy array. import numpy as np a = [] # declare null array a.append(['aa1']) # append elements a.append(['aa2']) a.append(['aa3']) print(a) a_np = np.asarray(a) # convert to numpy array print(a_np)
How do I declare an array in Python? - Stack Overflow
Oct 3, 2009 · @AndersonGreen As I said there's no such thing as a variable declaration in Python. You would create a multidimensional list by taking an empty list and putting other lists inside it or, if the dimensions of the list are known at write-time, you could just write it as a literal like this: my_2x2_list = [[a, b], [c, d]].
Declaring and populating 2D array in python - Stack Overflow
Mar 29, 2018 · The only time when you add 'rows' to the status array is before the outer for loop. So - status[0] exists but status[1] does not. you need to move status.append([]) to be inside the outer for loop and then it will create a new 'row' before you try to populate it.
python - 2d array of zeros - Stack Overflow
There is no array type in python, but to emulate it we can use lists. I want to have 2d array-like structure filled in with zeros. My question is: what is the difference, if any, in this two expressions: zeros = [[0 for i in xrange(M)] for j in xrange(M)] and. zeros = [[0]*M]*N
How to initialise a 2D array in Python? - Stack Overflow
Jun 3, 2014 · Declaring and populating 2D array in python. 2. declaring and initialising 2d array in python. 0.
How do I declare a 2d array in python? - Stack Overflow
Jan 20, 2018 · Example: the array is numbers[2,101] the first row is numbers 0 to 100 while the second is all empty strings. If the user input a number how can I search the array using their answer – Papi Harpy
2 dimensions array of unknown size in Python - Stack Overflow
Oct 17, 2013 · I am pretty new at python and numpy, so sorry if the question is kind of obvious. Looking on the internet I could not find the right answer. I need to create a 2 dimensions (a.ndim -->2) array of unknown size in python. Is it possible? I have found a way for 1 dimension passing through a list but no luck with 2 dimension. example
2D arrays in Python - Stack Overflow
In Python one would usually use lists for this purpose. Lists can be nested arbitrarily, thus allowing the creation of a 2D array. Not every sublist needs to be the same size, so that solves your other problem. Have a look at the examples I linked to.
How do I create an empty array and then append to it in NumPy?
To create an empty multidimensional array in NumPy (e.g. a 2D array m*n to store your matrix), in case you don't know m how many rows you will append and don't care about the computational cost Stephen Simmons mentioned (namely re-buildinging the array at each append), you can squeeze to 0 the dimension to which you want to append to: X = np.empty(shape=[0, n]).