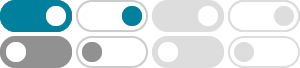
Bubble Sort - Python - GeeksforGeeks
Feb 21, 2025 · Bubble Sort is the simplest sorting algorithm that works by repeatedly swapping the adjacent elements if they are in the wrong order. Bubble Sort algorithm, sorts an array by repeatedly comparing adjacent elements and swapping them if they are in the wrong order.
Bubble Sort (With Code in Python/C++/Java/C) - Programiz
The bubble sort algorithm compares two adjacent elements and swaps them if they are not in the intended order. In this tutorial, we will learn about the working of the bubble sort algorithm along with its implementations in Python, Java and C/C++.
Python program for bubble sort [3 methods] - Python Guides
Oct 12, 2023 · In this Python article, I will explain how to write a Python program for bubble sort using different methods with some illustrative examples. Here, I will also explain what bubble sorting is, how bubble sort works in Python, and different ways to …
Bubble Sort in Python - AskPython
Feb 22, 2021 · Let’s study one of the most intuitive and easiest to learn sorting algorithms, and implement Bubble Sort in Python. We’ll start by understanding sorting itself, and then we’ll get to sorting via bubble sort, and finally, we’ll see how to implement it in Python.
Bubble Sort Algorithm - Python Examples
Bubble sort is a simple and intuitive sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. This process is repeated until the list is sorted.
Bubble Sort in Python (with code) - FavTutor
Apr 25, 2023 · Using the bubble sort algorithm to organize a list in Python is straightforward. Here is the code: for i in range (0, len (a_list) -1): . for j in range (len (a_list) -1): . if (a_list[j]> a_list[j +1]): . temp = a_list[j] a_list[j] = a_list[j +1] . a_list[j +1] = temp . …
Bubble Sort in Python - Example Project
Aug 11, 2023 · Dive into the world of bubble sort algorithm using Python. Explore a step-by-step guide to implement bubble sort with practical examples. Learn how to efficiently sort a list of elements in ascending order using this simple yet effective sorting technique.
Bubble Sort – Algorithm in Java, C++, Python with Example Code
Sep 29, 2022 · Here’s a code example showing the implementation of the bubble sort algorithm in Python: def bubble_sort (arr): arr_len = len(arr) for i in range(arr_len-1): flag = 0 for j in range(0, arr_len-i-1): if arr[j] > arr[j+ 1]: arr[j+ 1], arr[j] = arr[j], arr[j+ 1] flag = 1 if flag == 0: break return arr arr = [5, 3, 4, 1, 2] print("List sorted ...
Bubble Sort Program in Python - Sanfoundry
Write a Python program to implement bubble sort. n = len(lst) for i in range(n- 1): for j in range(n- 1 -i): if lst [j] > lst [j+ 1]: lst [j], lst [j+ 1] = lst [j+ 1], lst [j] In the code above, the bubble_sort function takes a list (lst) as input and performs the Bubble Sort algorithm.
Bubble Sort in Python: Program, Complexity, Working - Analytics …
Feb 2, 2024 · Below is a simple implementation of the Bubble Sort algorithm in Python: n = len (arr) for i in range (n): for j in range (0, n-i- 1): if arr[j] > arr[j+ 1]: arr[j], arr[j+ 1] = arr[j+ 1], arr[j] return arr. # Example usage . print (sorted_list) The time complexity of Bubble Sort is O (n^2), where n is the number of elements in the list.