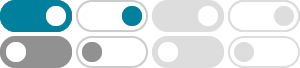
Binary Search (Recursive and Iterative) – Python - GeeksforGeeks
Feb 21, 2025 · Binary Search Algorithm is a searching algorithm used in a sorted array by repeatedly dividing the search interval in half. The idea of binary search is to use the information that the array is sorted and reduce the time complexity to O (log N). Below is the step-by-step algorithm for Binary Search:
Binary search (bisection) in Python - Stack Overflow
Oct 17, 2008 · While there's no explicit binary search algorithm in Python, there is a module - bisect - designed to find the insertion point for an element in a sorted list using a binary search.
Binary Search (bisect) in Python - GeeksforGeeks
Feb 4, 2022 · Binary Search is a technique used to search element in a sorted list. In this article, we will looking at library functions to do Binary Search. Finding first occurrence of an element. bisect.bisect_left (a, x, lo=0, hi=len (a)) : Returns leftmost insertion point of x in a sorted list.
Binary Search in Python
Binary search is a powerful algorithm that allows us to find a target value in a sorted list of items quickly and efficiently. By dividing the search space in half repeatedly, we can drastically reduce the number of comparisons we need to make, making it much faster than a linear search.
How to Do a Binary Search in Python
Binary search in Python can be performed using the built-in bisect module, which also helps with preserving a list in sorted order. It’s based on the bisection method for finding roots of functions.
Python Binary Search Algorithm: Efficient Search with Code …
Jan 23, 2025 · Learn how to implement the binary search algorithm in Python with step-by-step examples. Understand binary search time complexity, recursive and iterative methods, and real-world use cases to boost your coding skills.
Binary Search in Python (Recursive and Iterative)
Learn what is Binary Search Algorithm. Create Project for Binary Search Algorithm using Python modules like Tkinter for GUI.
Binary Search In Python
Mar 20, 2025 · In this article, I will explain binary search in Python with examples. Binary search is a divide-and-conquer algorithm that finds the position of a target value within a sorted array. Unlike linear search, which checks each element sequentially, binary search divides the search space in half with each step. Here’s why binary search matters:
Python Program for Binary Search (Recursive and Iterative)
Oct 6, 2019 · In this blog post, we will explore both recursive and iterative implementations of the binary search algorithm in Python. Additionally, we’ll provide detailed explanations of the logic behind each approach and showcase program outputs.
Binary Search Algorithm in Python: A Comprehensive Guide
Jan 26, 2025 · In Python, implementing this algorithm can significantly improve the performance of search operations, especially when dealing with large datasets. This blog post will explore the binary search algorithm in Python, covering its basic concepts, usage methods, common practices, and best practices.
- Some results have been removed