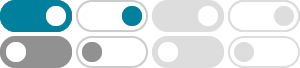
Converting array to Linked list - from Eloquent Javascript
reducer can be used to create linked list from array elements. function ListNode(val, next) { this.val = (val === undefined ? 0 : val) this.next = (next === undefined ? null : next) } let input = [1, 2, 3]; let head = input.reverse().reduce((acc, curr) => { if (acc == null) { acc = new ListNode(curr); } else { acc = new ListNode(curr, acc ...
Create linked list from a given array - GeeksforGeeks
Aug 1, 2024 · Given an array arr [] of size N. The task is to create linked list from the given array. Simple Approach: For each element of an array arr [] we create a node in a linked list and insert it at the end. Efficient Approach: We traverse array from end and insert every element at the beginning of the list. Time Complexity : O (n)
How to make a linked list from an array in Javascript
Mar 25, 2018 · If by constructor function you're talking about the linkedList(arr) {} function (which is more of a factory function), every call to it creates a new linked list since the list variable is local to the function and every function call has its own instance.
Implementation of LinkedList in Javascript - GeeksforGeeks
Mar 5, 2025 · To create a simple linked list in JavaScript, the provided code defines a LinkedList class and a Node class to represent individual elements. Node class: Each node holds a value and a reference (next) to the next node. LinkedList class: Manages the list, starting with an empty list (head is null).
How to Implement a Linked List in JavaScript - freeCodeCamp.org
Jun 2, 2020 · In this article, we will discuss what a linked list is, how it is different from an array, and how to implement it in JavaScript. Let's get started. What is a Linked List? A linked list is a linear data structure similar to an array. However, unlike arrays, elements are not stored in a particular memory location or index.
Are JavaScript arrays actually linked lists? - Stack Overflow
Aug 15, 2011 · Could Array be implemented on top of a linked list? Yes. This might make certain operations faster such as shift and unshift efficient, but Array also is frequently accessed by index which is not efficient with linked lists. It's also possible to get …
Create Linked List From A Given Array | Linked List | Prepbytes
Aug 3, 2021 · Learn to create a linked list in the simplest way from a given array. This blog explains how easily you can create a linked list from a given array of elements.
Create a Linked List From a given array - Naukri Code 360
May 8, 2024 · You are given an array nums [] of length n. The task is to create a linked list from the given array. The main idea of the approach is to traverse the array from the start and insert each element at the end of the linked list. Let’s see the algorithm step-by-step: Traverse the array and allocate memory for the new node for each element.
How to Implement a Linked List in JavaScript: An In-Depth Guide
Dec 8, 2024 · Based on teaching countless students, here is my proven step-by-step process for implementing a linked list in JavaScript: 1. Draw a Linked List Diagram. Visualizing how linked list nodes connect helps guide implementation. Here is a typical single-linked list diagram I …
JavaScript Data Structures: Arrays and Linked Lists
Nov 7, 2024 · To create a linked list in JavaScript, you can use an object to store the elements, and an array to keep track of the indices. For example: let LinkedList = function() {
- Some results have been removed