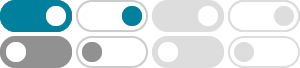
JavaScript Array push() Method - W3Schools
The push() method adds new items to the end of an array. The push() method changes the length of the array. The push() method returns the new length.
javascript - How to append something to an array ... - Stack Overflow
Dec 9, 2008 · To append a single item to an array, use the push() method provided by the Array object: const fruits = ['banana', 'pear', 'apple'] fruits.push('mango') console.log(fruits) push() mutates the original array. To create a new array instead, use the concat() Array method:
JavaScript Arrays - W3Schools
Using an array literal is the easiest way to create a JavaScript Array. Syntax: const array_name = [item1, item2, ...]; It is a common practice to declare arrays with the const keyword. Learn more about const with arrays in the chapter: JS Array Const. Spaces and line breaks are not important. A declaration can span multiple lines:
How to Add Elements to a JavaScript Array? - GeeksforGeeks
Nov 17, 2024 · Here are different ways to add elements to an array in JavaScript. 1. Using push () Method. The push () method adds one or more elements to the end of an array and returns the new length of the array. Syntax. 10, 20, 30, 40, 50, 60, 70. 2. Using unshift () Method.
How can I add new array elements at the beginning of an array in ...
If you want to push elements that are in an array at the beginning of your array, use <func>.apply(<this>, <Array of args>): const arr = [1, 2]; arr.unshift.apply(arr, [3, 4]); console.log(arr); // [3, 4, 1, 2]
JavaScript Add to an Array – JS Append - freeCodeCamp.org
Oct 14, 2022 · In this article, we talked about the different methods you can use to add and append elements to a JavaScript array. We gave examples using the push , splice , and concat methods as well as the ES6 spread syntax ( ...
JavaScript- Add an Object to JS Array - GeeksforGeeks
Jan 9, 2025 · These are the following ways to add an object to JS array: 1. Using JavaScript Array push () Method. The push () method is used to add one or multiple elements to the end of an array. It returns the new length of the array formed. An object can be inserted by passing the object as a parameter to this method. 2.
javascript - How to add an object to an array - Stack Overflow
Jun 6, 2011 · Put anything into an array using Array.push (). Extra information on Arrays. Add more than one item at a time. Add items to the beginning of an array. Add the contents of one array to another. Create a new array from the contents of two arrays.
How To Add New Elements To A JavaScript Array - W3docs
To add new elements you can use the following JavaScript functions: push () unshift (), concat () function or splice (). See examples.
How to Add and Remove Elements from Arrays in JavaScript
Mar 13, 2024 · In this article, you will learn how to work with the built in JavaScript methods: pop, push, shift and unshift. You will also learn how to work with the splice method which allows you to mutate the original array and add/remove elements at …