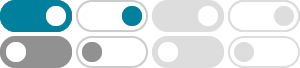
slice - How slicing in Python works - Stack Overflow
How Python Figures Out Missing Parameters: When slicing, if you leave out any parameter, Python tries to figure it out automatically. If you check the source code of CPython, you will find a function called PySlice_GetIndicesEx() which figures out indices to a slice for any given parameters. Here is the logical equivalent code in Python.
How do I declare an array in Python? - Stack Overflow
Oct 3, 2009 · @AndersonGreen As I said there's no such thing as a variable declaration in Python. You would create a multidimensional list by taking an empty list and putting other lists inside it or, if the dimensions of the list are known at write-time, you could just write it as a literal like this: my_2x2_list = [[a, b], [c, d]].
python - array.array versus numpy.array - Stack Overflow
Jun 21, 2022 · In defense of array.array, I think its important to note that it is also a lot more lightweight than numpy.array, and that saying 'will do just fine' for a 1D array should really be 'a lot faster, smaller, and works in pypy/cython without issues.'
How to declare and add items to an array in Python
Python's array module. The standard library also has the array module, which is a wrapper over C arrays. Like C arrays, array.array objects hold only a single type (which has to be specified at object creation time by using a type code), whereas Python list objects can hold anything.
How to create an integer array in Python? - Stack Overflow
Dec 7, 2009 · Use the array module. With it you can store collections of the same type efficiently. >>> import array >>> import itertools >>> a = array_of_signed_ints = array.array("i", itertools.repeat(0, 10)) For more information - e.g. different types, look at the documentation of the array module. For up to 1 million entries this should feel pretty snappy.
Python: How to get values of an array at certain index positions?
Aug 8, 2014 · It depends. If you already have a NumPy array, that should be faster. If you have to construct a NumPy array from your list first, the time it takes to do that and the selection may be slower than it would be to simply operate on the list. –
python - How to concatenate (join) items in a list to a single string ...
Sep 17, 2012 · This function was removed in Python 3 and Python 2 is dead. Even if you are still using Python 2 you should write Python 3 ready code to make the inevitable upgrade easier. Although @Burhan Khalid's answer is good, I think it's more understandable like this:
Python: find position of element in array - Stack Overflow
For your first question, find the position of some value in a list x using index(), like so:. x.index(value) For your second question, to check for multiple same values you should split your list into chunks and use the same logic from above.
Python array elements in if statement - Stack Overflow
Apr 24, 2018 · I have some array with integers, and for loop. I am trying to test if some specific elements in array is bigger or smaller that some integer. This code explain it better: array = [1,2,3,4,5] for i...
How do I compute the derivative of an array in python
May 30, 2013 · ) Same shape-size as input array. Uses second order accurate central differences in the interior points and either first or second order accurate one-sides (forward or backwards) differences at the boundaries. The returned gradient hence has the same shape as the input array. 2. Use numpy.diff (you probably don't want this)