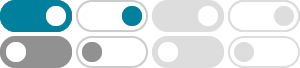
Python reversing a string using recursion - Stack Overflow
I want to use recursion to reverse a string in python so it displays the characters backwards (i.e "Hello" will become "olleh"/"o l l e h". I wrote one that does it iteratively: result = "" n = 0. start = 0. while ( s[n:] != "" ): while ( s[n:] != "" and s[n] != ' ' ): n = n + 1. result = s[ start: n ] + " " + result. start = n. return result.
How do I reverse a list using recursion in Python?
Oct 19, 2008 · I want to have a function that will return the reverse of a list that it is given -- using recursion. How can I do that? A bit more explicit: if len(l) == 0: return [] return [l[-1]] + rev(l[:-1]) This turns into: if not l: return [] return [l[-1]] + rev(l[:-1]) Which turns into: return [l[ …
Print reverse of a string using recursion - GeeksforGeeks
Mar 6, 2025 · # Python program to reverse a string def reverse (str, start, end): if start >= end: return str [start], str [end] = str [end], str [start] # Swap characters reverse (str, start + 1, end-1) if __name__ == '__main__': str = list ("Geeks for Geeks") reverse …
Reversing a list using recursion in python - Stack Overflow
Recursive case is that you do, so you want to prepend the last element to the recursive call on the rest of the list. def revlist(lst): if not lst: return lst # Create a list containing only the last element last = [lst[-1]] # Rest contains all elements up to the last element in `lst` rest = lst[:-1] # Prepend last to recursive call on `rest ...
Reverse a String Using Recursion in Python - Online Tutorials …
Mar 12, 2021 · Learn how to reverse a string using recursion in Python with this step-by-step guide and example.
Python Reverse String – Recursive String Reversal Explained And ...
Sep 3, 2024 · Step-by-step walkthrough of reversing strings recursively ; Python implementation of recursive string reversal code; Explanation of the recursion call stack ; Tradeoffs between recursion and iterative alternatives; Optimization and limitations when applying recursion in Python; Let’s start by solidifying what recursion is and why it’s used.
5 Best Ways to Reverse a String in Python Using Recursion
Mar 7, 2024 · This article demonstrates five recursive methods to achieve this in Python. Method 1: Basic Recursion. One straightforward approach to reverse a string using recursion involves creating a function that concatenates the last character of the string with a recursive call that excludes this character.
Python String Reversal
String reversal is a common programming task that can be approached in multiple ways in Python. This comprehensive guide will explore various methods to reverse a string, discussing their pros, cons, and performance characteristics.
How to Reverse a list using recursion in Python
To reverse a list using recursion we use a two-pointer approach. In this approach, we take two pointers L & R initially L point to the first element of the list and R points to the last element of the list. Let’s understand whole concepts with the help of an example.
Reversing a String In Python Using Recursion | by Fridah - Medium
Aug 8, 2023 · If the input string is not empty, the function makes a recursive call to reversing_string() with the argument string[1:]. This slices the input string from the second character onward and...
- Some results have been removed