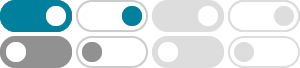
Best way in javascript to repeatedly repeat a function?
Apr 25, 2014 · To use set time out, you need to put the function in but not call it. Like so: setTimeout(hundredSteps, 10) or. setTimeout(function() { hundredSteps() }, 10) I would clean it all up a bit, for example. In your question you have stage being incremented with counter, when it should be incremented in the next step function.
javascript - Calling a function every 60 seconds - Stack Overflow
Oct 27, 2017 · JavaScript: get code to run every minute. If you don't care if the code within the timer may take longer than your interval, use setInterval(): That fires the function passed in as first parameter over and over. A better approach is, to use setTimeout along with a self-executing anonymous function: // do some stuff.
JavaScript String repeat() Method - W3Schools
The repeat() method returns a string with a number of copies of a string. The repeat() method returns a new string. The repeat() method does not change the original string.
Repeat a string in JavaScript a number of times - Stack Overflow
Jul 5, 2024 · The following function will perform a lot faster than the option suggested in the accepted answer: var repeat = function(str, count) { var array = []; for(var i = 0; i < count;) array[i++] = str; return array.join(''); } You'd use it like this : var repeatedString = repeat("a", 10);
Automatically Repeat JavaScript Function Every X Seconds
Sep 26, 2021 · In JavaScript, we can use the built-in setInterval() function to repeat any given function over a certain interval: console. log (`Hello world!`); setInterval (myFunction, 2000); // Repeat myFunction every 2 seconds. setInterval() takes two parameters.
Repeatedly call a function in JavaScript | Techie Delight
Apr 17, 2024 · This post will discuss how to repeatedly call a function or execute the specified code in JavaScript. 1. Using setInterval() method. The following solution uses the setInterval () method to repeatedly call a function with a specified time delay between each call.
How to Implement JavaScript Auto-Repeat Functionality
Feb 8, 2023 · Here is an example of how to implement an auto-repeat function in JavaScript: console.log('Action repeated.'); repeatAction(); setTimeout(startAutoRepeat, 500); In this example, the repeatAction() function represents the action that you want to repeat.
String.prototype.repeat() - JavaScript | MDN - MDN Web Docs
Mar 13, 2025 · The repeat () method of String values constructs and returns a new string which contains the specified number of copies of this string, concatenated together.
JavaScript: Repeat a function X times at I intervals
Jun 25, 2012 · I've written a general purpose repeat function which allows you to repeat a callback function X times separated by I intervals with the option to start immediately or after the interval. It can also default to just looping infinitely, which is what setInterval does.
Javascript repeat a function x amount of times - Stack Overflow
You may not be able to eval() a function, but you can call it. Here's the fix: function repeat(func, times) { for (x = 0; x < times; x++) { func() } }