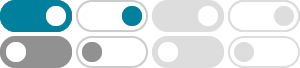
printing a two dimensional array in python - Stack Overflow
Jun 4, 2017 · import numpy as np def printArray(args): print "\t".join(args) n = 10 Array = np.zeros(shape=(n,n)).astype('int') for row in Array: printArray([str(x) for x in row]) If you want to only print certain indices:
How to Create a 2D NumPy Array in Python? - Python Guides
Nov 20, 2023 · Methods to create a 2D NumPy array in Python. Method 1: np 2d array in Python with the np.array() function. Method 2: Create a 2d NumPy array using np.zeros() function; Method 3: NumPy 2D array initialize using np.ones() function; Method 4: How to create a 2d NumPy array using np.full() function
python - Pretty print 2D list? - Stack Overflow
Is there a simple, built-in way to print a 2D Python list as a 2D matrix? So this: would become something like. I found the pprint module, but it doesn't seem to do what I want. I would have called that a 3D list. If you are willing to pull it in, numpy is pretty good about this sort of thing.
how to plot two-dimension array in python? - Stack Overflow
Mar 27, 2015 · 1) Python does not have the 2D, f[i,j], index notation, but to get that you can use numpy. Picking a arbitrary index pair from your example: import numpy as np f = np.array(data) print f[1,2] # 6 print data[1][2] # 6 2) Then for the plot you can do: plt.imshow(f, interpolation="nearest", origin="upper") plt.colorbar() plt.show()
How to Print an Array in Python - AskPython
Mar 30, 2020 · Similar to the case of arrays implemented using lists, we can directly pass NumPy array name to the print() method to print the arrays. import numpy as np arr_2d = np.array([[21,43],[22,55],[53,86]]) arr = np.array([1,2,3,4]) print("Numpy array is: ", arr) #printing the 1d numpy array print("Numpy 2D-array is: ", arr_2d) #printing the 2d numpy ...
Create 2D Array in NumPy - Python Examples
Learn how to create a 2D array in Python using NumPy. Explore various methods like array(), zeros(), ones(), and empty() to easily initialize 2D arrays with different values and shapes.
Python | Using 2D arrays/lists the right way - GeeksforGeeks
Jun 20, 2024 · In this article, we will explore the right way to use 2D arrays/lists in Python. Using 2D arrays/lists the right way involves understanding the structure, accessing elements, and efficiently manipulating data in a two-dimensional grid. When working with structured data or grids, 2D arrays or lists can be useful.
NumPy Array in Python - GeeksforGeeks
Jan 24, 2025 · In this article, we will explore NumPy Array in Python. Create NumPy Arrays. To start using NumPy, import it as follows: NumPy array’s objects allow us to work with arrays in Python. The array object is called ndarray. NumPy arrays are created using the array () function. Example: [3 4]] [[5 6] [7 8]]] Key Attributes of NumPy Arrays.
Examples of Generating 2-D Numpy Array - tidystat.com
Jun 11, 2022 · This tutorial provides 3 methods to create 2-D Numpy Arrays in Python. The following is the key syntax for these 3 methods. After that, 3 Python Numpy code examples are provided. Method 1: np.array[[numbers in the first row ], [numbers in the second row]] Method 2: np.zeros(shape(row number, column number)) Method 3: array_name=np.arange(length ...
Multidimensional Arrays in Python: A Complete Guide
Feb 27, 2023 · Let’s start with implementing a 2 dimensional array using the numpy array method. arr = np.array([array1 values..][array2 values...]) [5,6,7,8]]) OUTPUT. A 3-D (three-dimensional) array is mainly composed of an array of 2-D arrays. The rows, columns, and page elements can be viewed as primary components of a 3D array.