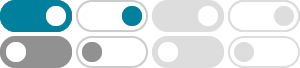
python - How to repeatedly execute a function every x seconds?
This code will run as a daemon and is effectively like calling the python script every minute using a cron, but without requiring that to be set up by the user. In this question about a cron implemented in Python , the solution appears to effectively just sleep() for x seconds.
python - How to call a script from another script? - Stack Overflow
As I found this, when I was searching for a solution in python 3. In python 3 you can just use the importfunctions (note that the py.files should not have a name as a library, it should have a unique name): File test1.py: print "I am a test" print "see! I do …
How do I execute a program or call a system command?
Note on Python version: If you are still using Python 2, subprocess.call works in a similar way. ProTip: shlex.split can help you to parse the command for run, call, and other subprocess functions in case you don't want (or you can't!) provide them in form of lists: import shlex import subprocess subprocess.run(shlex.split('ls -l'))
How to execute a file within the Python interpreter?
Nov 6, 2023 · if your code is mycode.py for instance, and you type just 'import mycode', Python will execute it but it will not make all your variables available to the interpreter. I found that you should type actually 'from mycode import *' if you want to make all variables available to …
Possible to execute Python bytecode from a script?
Apr 27, 2015 · Of course one could just compress the code too, but smaller byte-code may be harder to detect. When auditing for malicious Python code, its useful to know what options are available and what malicious commands may look like. Further there would be some legitimate uses for this too. (where the overhead of compiling on the client is noticeable).
How do I execute a string containing Python code in Python?
I wanted to execute specific code for different users/customers but also wanted to avoid the exec/eval. I initially looked to storing the code in a database for each user and doing the above. I ended up creating the files on the file system within a 'customer_filters' folder and using the 'imp' module, if no filter applied for that customer, it ...
python - Doing something before program exit - Stack Overflow
May 3, 2014 · @RacecaR, the only way you can run termination code even if a process badly crashes or is brutally killed is in another process, known as a "monitor" or "watchdog", whose only job is to keep an eye on the target process and run the termination code when apropriate. Of course that requires a very different architecture and has its limitations ...
python - Run function from the command line - Stack Overflow
This allows you to execute the script simply by running python myfile.py or python -m myfile. Some explanation here: __name__ is a special Python variable that holds the name of the module currently being executed, except when the module is started from the command line, in which case it becomes "__main__".
How do I find/excute Python Interactive Mode in Visual Studio …
Nov 7, 2020 · F1 → Python: Create Python Interactive Window. This is the Python interactive window that comes with Visual Studio Code. You can enter the interactive window without inputting instructions. Please use "Ctrl+Enter" to execute the code: Option 2. Ctrl + Shift + ` This is the cmd window that comes with the Visual Studio Code integration computer.
Efficient way of having a function only execute once in a loop
Jun 6, 2014 · Another option is to set the func_code code object for your function to be a code object for a function that does nothing. This should be done at the end of your function body. For example: def run_once(): # Code for something you only want to execute once run_once.func_code = (lambda:None).func_code